Python provides us with various ways to retrieve the last element in a list. These methods include:
- Method 1: Using Negative Indexing
- Method 2: Using len() Function
- Method 3: Using pop() Method
- Method 4: Using reversed() and next() Function
Let’s break down each of these methods!
Method 1: Getting the Last Element in a List Using Negative Indexing
In Python, negative indexing allows programmers to access sequence elements from the end. It starts from “-1” and goes backward. We can make use of this method to get the last element in a list.
Let’s see how:
- First of all, define a list with some values.
- Get the last element by passing “-1” as the index and store it in a variable.
- Lastly, print it.
names = ["Karin", "Asen", "Elsa", "John", "Fin"]
last_element = names[-1]
print("Last Element: "+last_element)
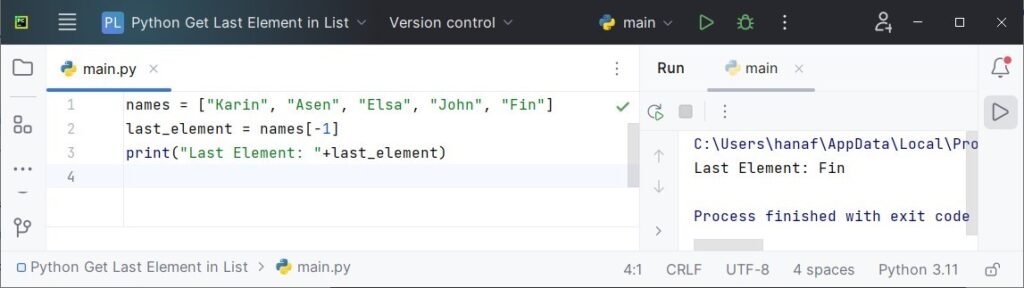
Method 2: Getting the Last Element in a List Using len() Function
The len() function returns the length of an object. The object can either be a sequence or a collection.
To use this function to the last element in a list, follow:
- Create a list with a few string values.
- Use the len() function to find out the length of the list, subtract 1 from it. Then, retrieve and print the element at this index.
- After that, store the value in a variable and print it.
names = ["Karin", "Asen", "Elsa", "John", "Fin"]
last_element = names[len(names) - 1]
print("Last Element: "+last_element)
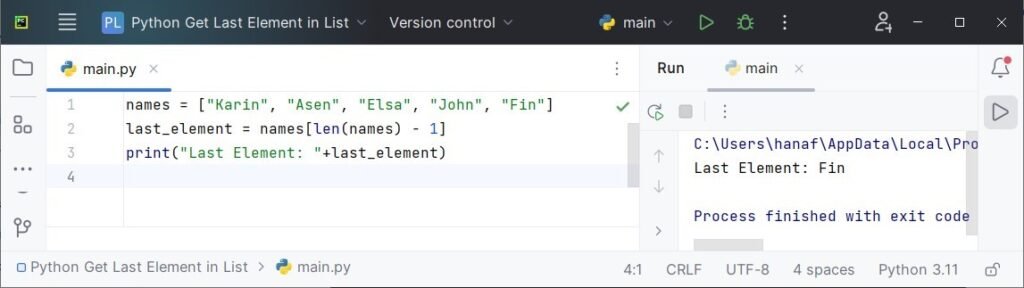
Method 3: Getting the Last Element in a List Using pop() Method
In Python, if no index is provided, the pop() method discards the last element from the list.
Here is how you can use it to get the last element in a list:
- Create a list and assign it some values.
- Call the pop() method on the list and print the result.
- Lastly, print the list as well to see the difference.
names = ["Karin", "Asen", "Elsa", "John", "Fin"]
last_element = names.pop()
print("Last Element: "+last_element)
print(names)
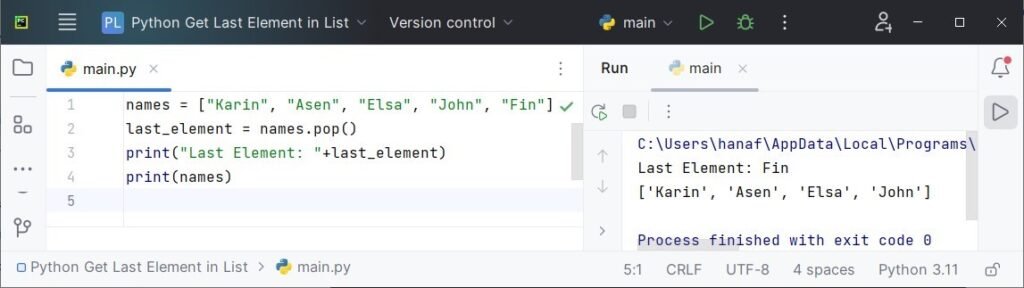
Method 4: Getting the Last Element Using reversed() and next() Functions
The reversed() function takes an iterable and returns its reversed version. Whereas, the next() function returns the next element from an iterator.
Both of these can be utilized to get the last element in a list in Python:
- Create a list holding some values.
- Apply the reversed() function on the list and pass the result to the next() function.
- Calling the next() function for the first time will return the first element of the reversed list.
names = ["Karin", "Asen", "Elsa", "John", "Fin"]
last_element = next(reversed(names))
print("Last Element: "+last_element)
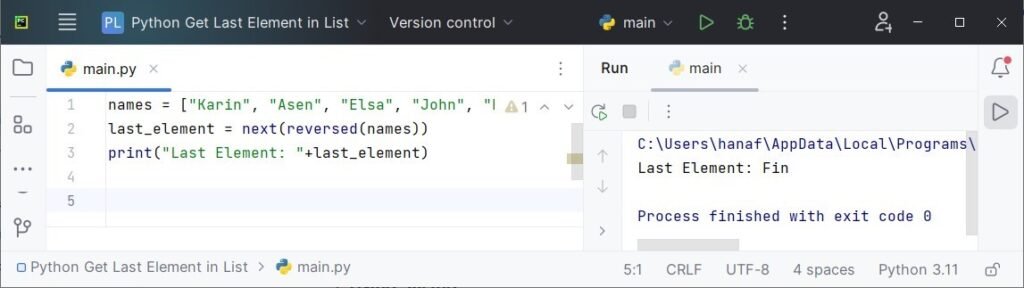
Conclusion
There are various ways to get the last element in a list in Python. This includes using negative indexing, the len() function, the pop() method, and the reverse() function along with the next() function. Go through the blog to see how they work.