If you are a beginner and working on math skills, knowing how to square a number in Python is essential.
In this guide, we’ll walk you through several methods to achieve square a number in Python.
How to Square a Number in Python?
Squaring a number in Python is a fundamental operation that can be achieved in several ways, each with its own advantages.
To square a number in Python, use the below methods:
Method 1: Using the Exponentiation Operator
The easiest way to square a number in Python is through the exponentiation operator (**). It raises the particular number to the power of another particular number.
To square a number, simply raise it to the power of 2 as below:
number = 5
squared = number ** 2
print(squared) # Output: 25
In this example, number ** 2 calculates 5 raised to the power of 2, resulting in 25. This method is quick and easy, making it a favorite among Python programmers.
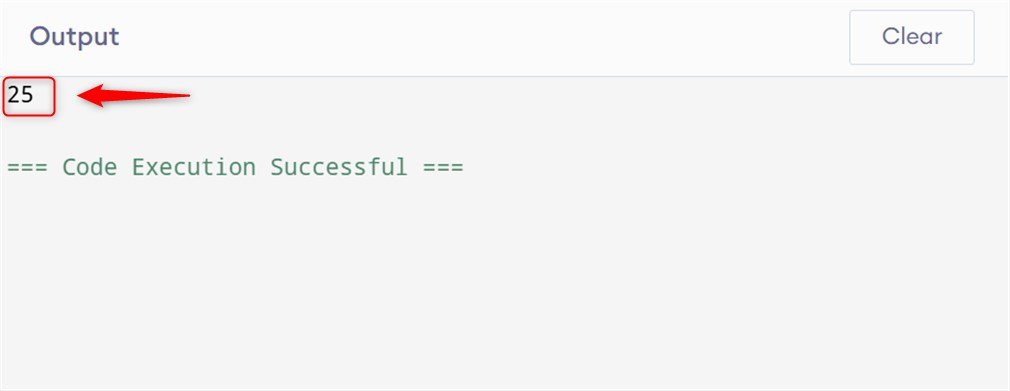
Method 2: Using the pow() Function
Another method to square the number is possible through the built-in pow() function. It takes two parameters, one is the base and the second one is the exponent.
To do so, you pass the number 10 as the base and 2 as the exponent.
number = 10
squared = pow(number, 2)
print(squared) # Output: 100
The pow() function is versatile and can be used for more complex mathematical operations, but it’s just as effective for simple squaring.
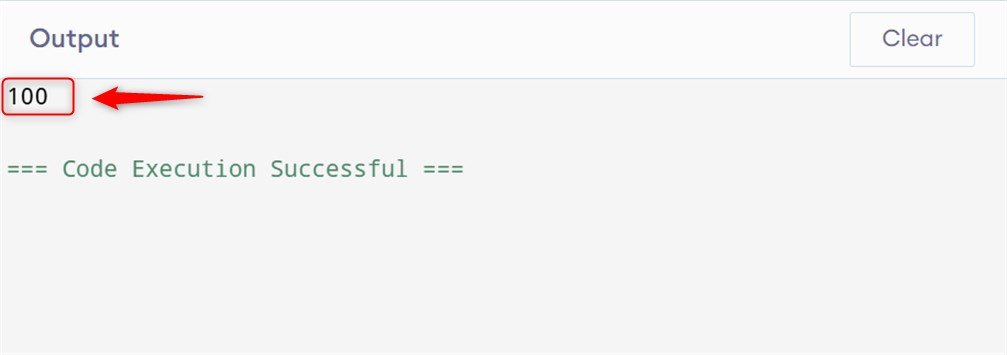
Method 3: Using Multiplication
If you prefer a more intuitive approach, you can simply multiply the number by itself. This method is straightforward and easy to understand, especially for those new to programming.
Let’s square the number 2 in Python:
number = 2
squared = number * number
print(squared) # Output: 4
Multiplying the number by itself is a clear and direct way to achieve the same result as the previous methods.
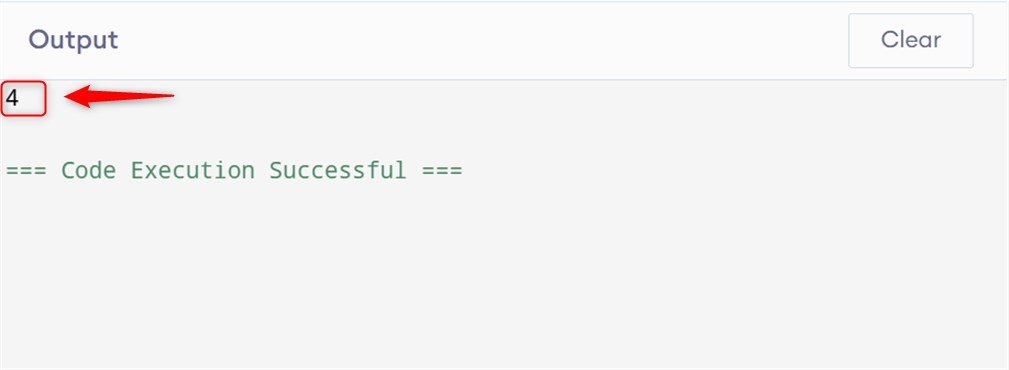
Method 4: Using NumPy Library
For those working with large datasets or performing complex mathematical operations, the NumPy library offers a powerful and efficient way to square numbers.
First, install NumPy on the system if you haven’t already:
pip install numpy
Once installed, you can use the numpy.square() function to square a number such as 5:
import numpy as np
number = 5
squared = np.square(number)
print(squared) # Output: 25
It is useful for scientific computing and data analysis, making it a valuable tool for more advanced Python users.
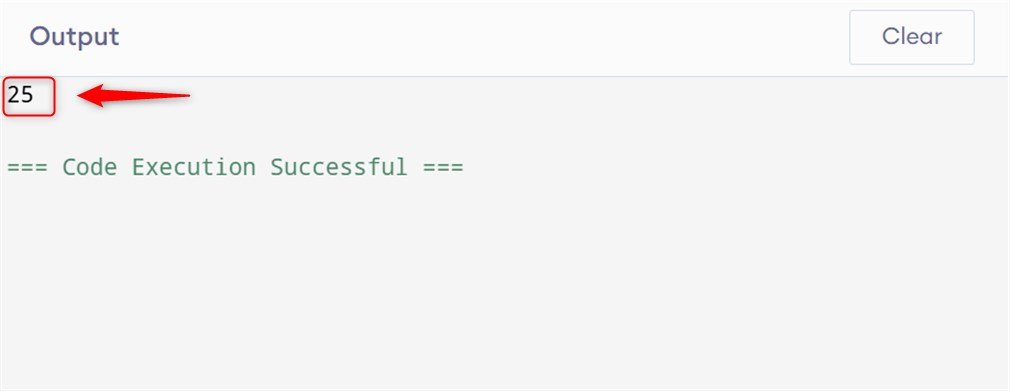
That is all from this guide.
Conclusion
To square a number in Python, you can use the exponentiation operator (**) like this: number ** 2, or simply multiply the number by itself: number * number. Both methods give you the squared value.
Whether you prefer the simplicity of the exponentiation operator, the versatility of the pow() function, the intuitiveness of multiplication, or the power of the NumPy library, this guide has covered all.