Are you worried and thinking about getting the index of the key in the Python dictionary? This guide is for you.
Python dictionaries are powerful data structures. They allow you to store and retrieve data efficiently using key-value pairs. However, there may be times when you need to find the index of a key within a dictionary.
This article will demonstrate several methods for getting the key index in a Python dictionary.
How to Get the Index of Key in Python Dictionary
Getting the index of a key in a Python dictionary is a common task that can be accomplished in several ways. At the same time, dictionaries themselves do not maintain order or indices.
You can achieve this by converting the dictionary keys to a list via the below methods:
Method 1: Using the list() Function
In a Python dictionary, you can use the list() method to convert the dictionary keys to a list. After that, you can obtain the key index through the index() method.
Here’s how you can do it:
my_dict = {'a': 1, 'b': 2, 'c': 3}
key_to_find = 'b'
keys_list = list(my_dict.keys())
index = keys_list.index(key_to_find)
print(f"The index of the key '{key_to_find}' is: {index}")
This code snippet converts the keys of a dictionary to a list and finds the index of a specified key. It then prints the index of the key ‘b‘, which is 1 in this case. This method is straightforward and works well for small to medium-sized dictionaries.
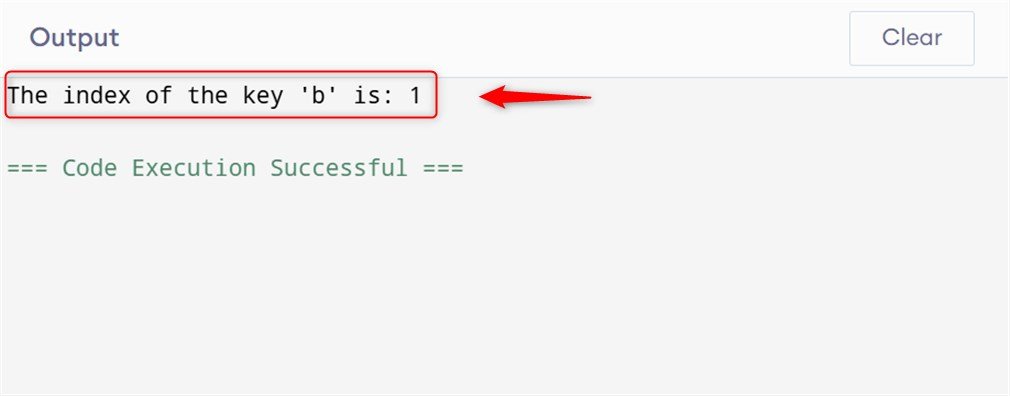
Method 2: Using a Loop
For obtaining the index of a key in a Python dictionary, you can utilize the loop. It iterates through the dictionary keys. This method is useful if you prefer a more manual approach or need to perform additional operations during the iteration.
my_dict = {'a': 1, 'b': 2, 'c': 3}
key_to_find = 'c'
index = -1
for i, key in enumerate(my_dict.keys()):
if key == key_to_find:
index = i
break
print(f"The index of the key '{key_to_find}' is: {index}")
Here, first, use the enumerate() function to get both the index as well as the key. Then, find the particular key store its index, and break out of the loop.
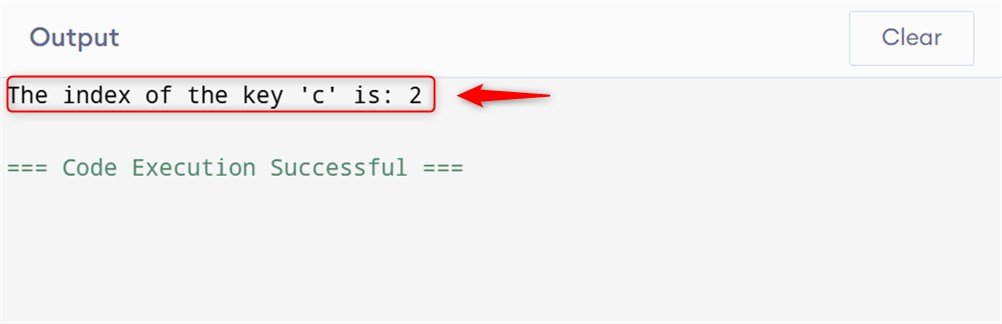
Method 3: Using List Comprehension
List comprehension provides a concise way to get the indexing of a key in a Python dictionary. This method combines the conversion of dictionary keys to a list with the index() method in a single line of code.
my_dict = {'a': 1, 'b': 2, 'c': 3}
key_to_find = 'b'
index = [i for i, key in enumerate(my_dict.keys()) if key == key_to_find][0]
print(f"The index of the key '{key_to_find}' is: {index}")
In this example, we use list comprehension to create a list of indices where the key matches the specified key. Since we expect only one match, we access the first element of the resulting list to get the index.
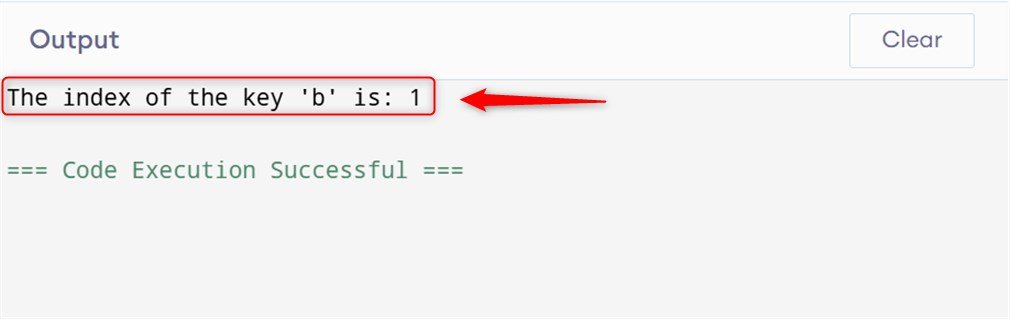
Method 4: Using the next() Function
In Python, the next() function is used in combination with a generator expression to get the key index. This method is efficient and avoids creating an intermediate list.
my_dict = {'a': 1, 'b': 2, 'c': 3}
key_to_find = 'b'
index = next((i for i, key in enumerate(my_dict.keys()) if key == key_to_find), -1)
print(f"The index of the key '{key_to_find}' is: {index}")
In this example, we use a generator expression to find the index of the specified key. The next() function returns the first matching index or -1 if the key is not found.
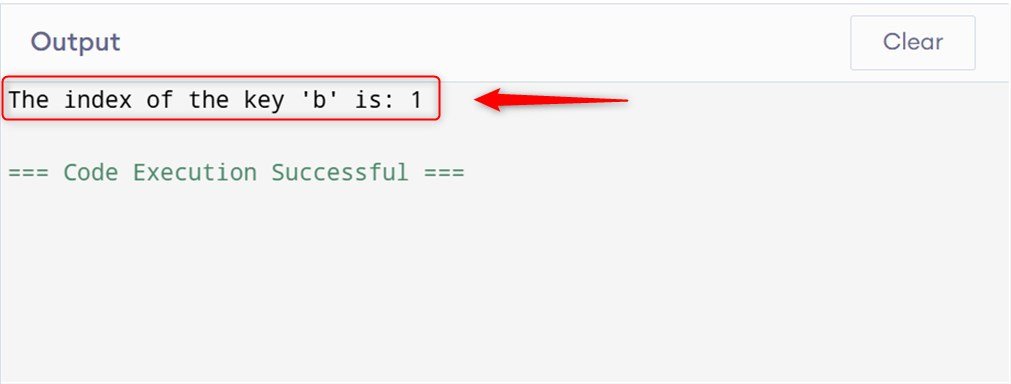
That is all from this guide.
Conclusion
To obtain the index of a key in a Python dictionary, first, convert the dictionary keys to a list using list(my_dict.keys()), and then use the index() function as follows: keys_list.index(key_to_find).