If you are looking to use tuple type hints in Python, it’ss the right place to answer this query!
One of the powerful features of type hinting is the ability to use tuples, which are immutable sequences often used to group related data. Python’s type hinting system has become an essential tool for developers. It provides a way to specify the expected types of variables, function arguments, and return values.
This guide will explore how to use tuple type hints in Python.
Understanding Tuples and Type Hints
Tuples are a fundamental data structure in Python, used to store a fixed collection of items.
Type hints for tuples allow you to specify the types of elements within the tuple. It provides better code readability and helps with static type checking.
Using tuple type hints in Python is a powerful way to enhance code readability and maintainability. Let’s use it:
Basic Tuple Type Hints
To specify the type of a tuple, you can use the Tuple type from the typing module. For example, if you have a tuple containing an integer and a string, you can specify its type as follows:
from typing import Tuple
def process_data(data: Tuple[int, str]) -> None:
print(f"ID: {data[0]}, Name: {data[1]}")
data = (123, "John")
process_data(data)
In the above code, the process_data function expects a tuple having an integer and a string. The type hint Tuple[int, str] clearly indicates the expected types of the tuple elements.
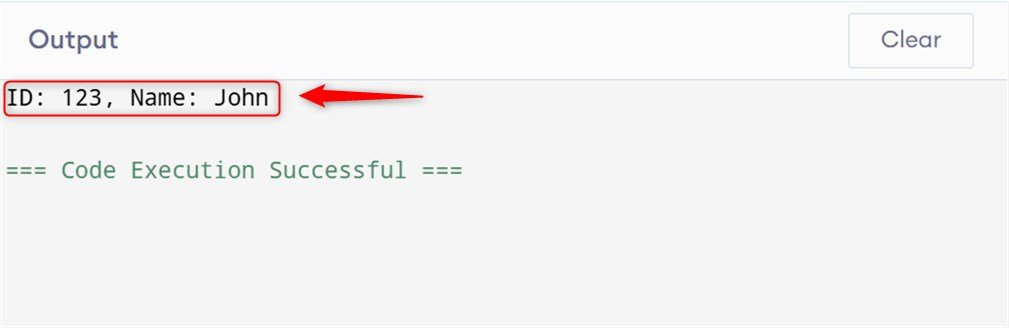
Variable-Length Tuples
Sometimes, you may need to work with tuples of variable length, where the number of elements is not fixed. You can use the ellipsis (…) to indicate that the tuple can contain any number of elements of a specified type.
Let’s see an example below:
from typing import Tuple
def sum_numbers(numbers: Tuple[int, ...]) -> int:
return sum(numbers)
numbers = (1, 2, 3, 4, 5)
print(sum_numbers(numbers))
Here, the sum_numbers function expects a tuple holding any number of integers. The type hint Tuple[int, …] indicates that the tuple can have a variable length, with all elements being integers.
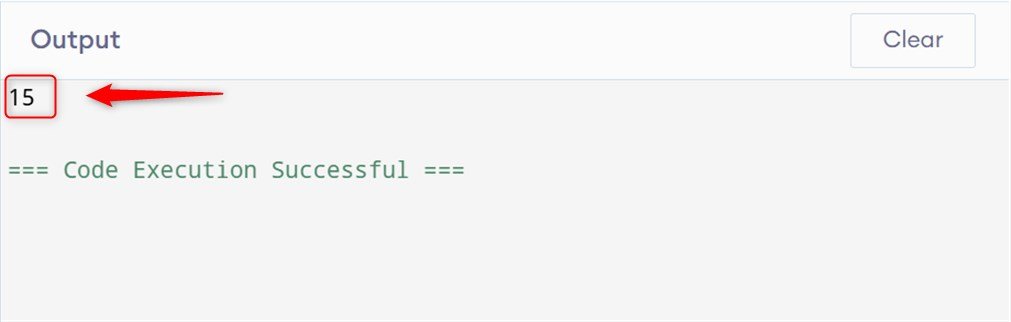
Nested Tuples
Tuples can also be used as nested (tuples within tuples). Type hints for nested tuples can be specified by nesting the Tuple type hints:
from typing import Tuple
def process_nested_data(data: Tuple[int, Tuple[str, float]]) -> None:
print(f"ID: {data[0]}, Info: {data[1][0]}, Score: {data[1][1]}")
data = (123, ("Henry", 95.5))
process_nested_data(data)
In this example, the process_nested_data function expects a tuple containing an integer and another tuple with a string and a float. The type hint Tuple[int, Tuple[str, float]] clearly indicates the structure and types of the nested tuple elements.
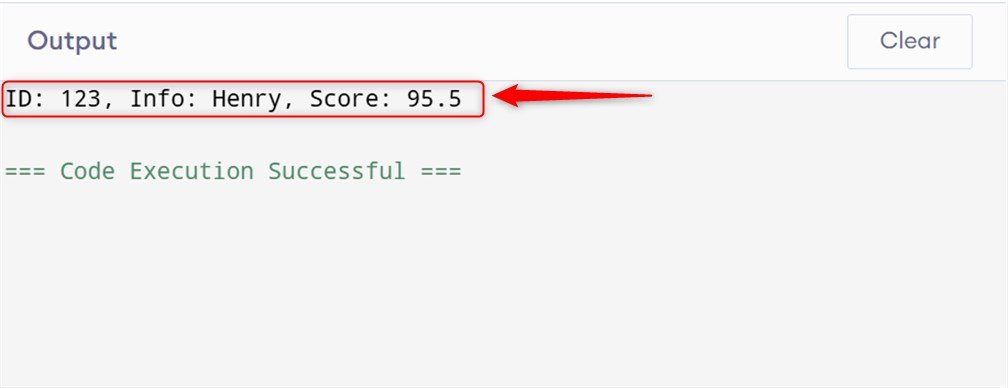
That is all from this guide.
Conclusion
Python’s type hinting system provides the flexibility you need to write robust and reliable code whether you’re working with basic tuples, variable-length tuples, or nested tuples.
To use tuple type hints in Python, import Tuple from the typing module and specify the types of the elements within the tuple, like this: Tuple[int, str]. This enhances code readability and helps with static type checking.