If you are worrying about checking if a field has changed in Python, we are here to fix it!
In the world of software development, tracking changes in data is a common task. Whether you’re working on a web application, a data processing script, or any other project, knowing how to check if a field has changed can be incredibly useful.
In this guide, we’ll explore different methods to check if a field has changed in Python.
How to Check if a Field Has Changed in Python
Before we dive into the how-to, let’s understand why checking for field changes is important:
- Data Integrity: Ensuring that data remains consistent and accurate.
- Triggering Actions: Performing specific actions when data changes, such as updating a database or sending notifications.
- Version Control: Keeping track of changes for auditing and debugging purposes.
Method 1: Using Variables
One of the simplest ways to check if a field has changed is by using variables to store the old and new values.
Here’s an example:
old_value = "apple"
new_value = "banana"
if old_value != new_value:
print("The field has changed.")
else:
print("The field has not changed.")
In this example, we compare the older values with the newer values of the field. If they are different, we print a message indicating that the field has changed.
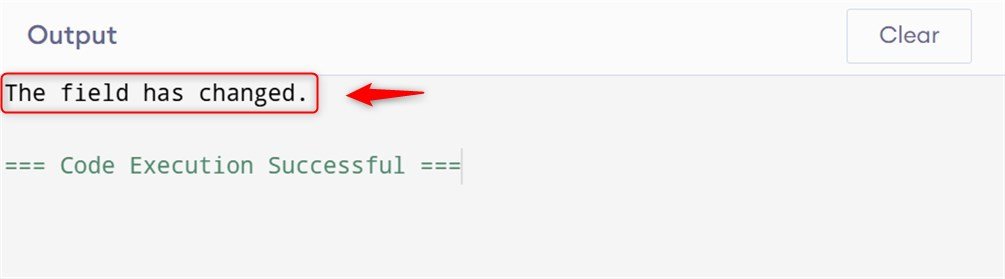
Method 2: Using Dictionaries
If you’re working with multiple fields, using dictionaries can be a more efficient approach.
Here’s an example:
old_data = {"name": "Alice", "age": 30}
new_data = {"name": "Alice", "age": 31}
for key in old_data:
if old_data[key] != new_data[key]:
print(f"The field '{key}' has changed from {old_data[key]} to {new_data[key]}.")
In this example, we iterate through the keys in the dictionary and compare the old and new values for each field. If a field has changed, we print a message indicating the change.
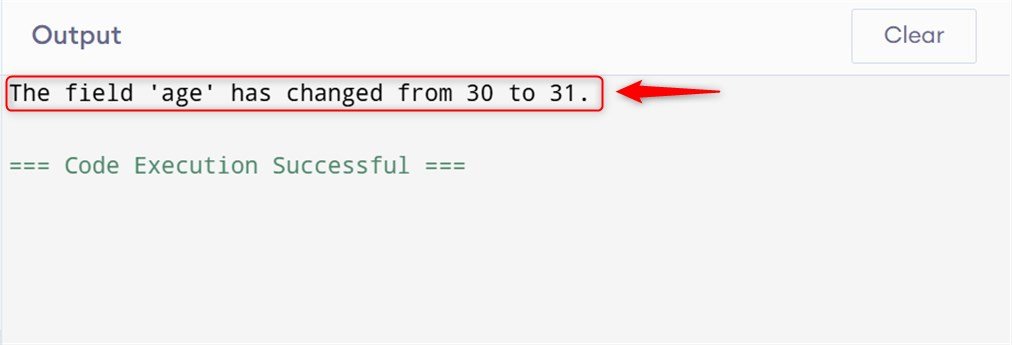
Method 3: Using Classes and Properties
For more complex scenarios, using classes and properties can provide a more structured approach.
Here’s an example:
class Person:
def __init__(self, name, age):
self._name = name
self._age = age
self._old_age = age
@property
def age(self):
return self._age
@age.setter
def age(self, value):
if self._age != value:
print(f"The field 'age' has changed from {self._age} to {value}.")
self._old_age = self._age
self._age = value
person = Person("Alice", 30)
person.age = 31 # This will trigger the change detection
In this example, we define a Person class with a property for the age field. The setter method for the age property checks if the value has changed and prints a message if it has.
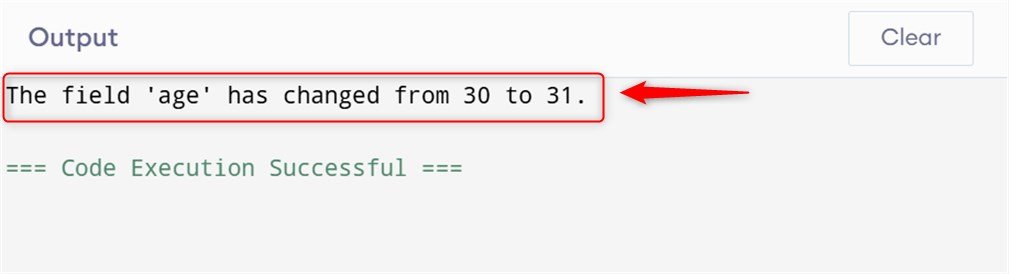
That is all from this guide.
Conclusion
Checking if a field has changed in Python is a common task that can be accomplished using various methods, from simple variable comparisons to more complex class properties and database queries. By understanding and implementing these techniques, you can ensure data integrity, trigger actions based on changes, and maintain version control in your projects.
Whether you’re working on a small script or a large application, these methods will help you manage data changes efficiently.