If you are a beginner or an experienced programmer, knowing how to add a dictionary in Python is an essential skill.
Python dictionaries are robust data structures that let you use key-value pairs to store and retrieve data.
This guide will explore several methods to add to a dictionary in Python.
How To Add to a Dictionary in Python
Adding elements to a dictionary in Python is a common task that can be accomplished in several ways.
Let’s explore one by one:
Method 1: Using the Assignment Operator
The simplest and most common way to add a new key-value pair to a dictionary is to use the assignment operator (=).
Let’s see an example:
my_dict = {'a': 1, 'b': 2}
my_dict['c'] = 3
print(my_dict)
Here, first of all, add the entry ‘c‘ with value 3 to the my_dict dictionary. Next, the new key-value pair is added to the dictionary.
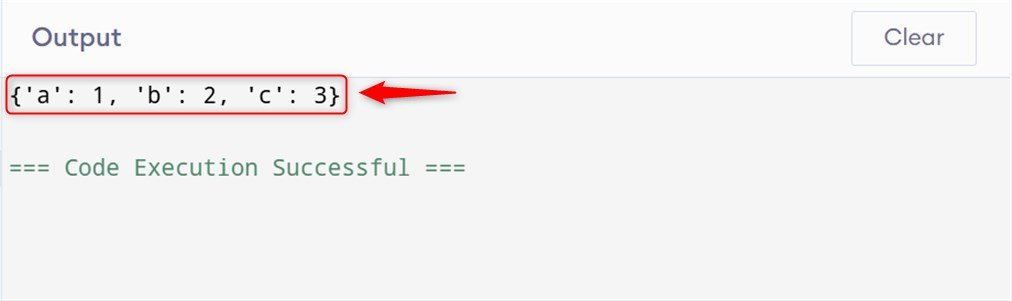
Method 2: Using the update() Method
For adding more than one key-value pair to a dictionary, use the update() method. This method is particularly useful when you need to merge another dictionary or add several elements simultaneously.
Let’s see the example below:
my_dict = {'a': 1, 'b': 2}
new_elements = {'c': 3, 'd': 4}
my_dict.update(new_elements)
print(my_dict)
Here, the update() method adds the key-value pairs from new_elements to my_dict. After that, the dictionary is modified to add new elements.

Method 3: Using Dictionary Comprehension
To create and update dictionaries, use Dictionary comprehension. This method is used to expand an existing dictionary by adding additional key-value pairs.
Let’s see an example:
my_dict = {'a': 1, 'b': 2}
new_elements = {'c': 3, 'd': 4}
my_dict = {**my_dict, **new_elements}
print(my_dict)
In this example, we use dictionary comprehension to merge my_dict and new_elements into a new dictionary. The original dictionary is then updated to include the new elements.
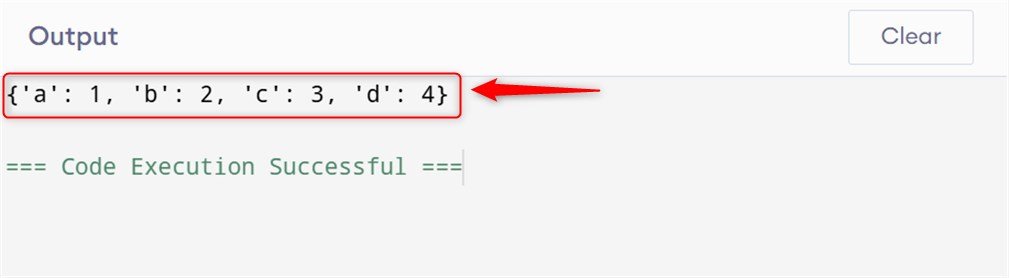
Method 4: Using the setdefault() Method
If the key does not already exist, the setdefault() method adds a key-value pair to a dictionary only. This method is useful when you want to ensure that a key is present in the dictionary without overwriting its value if it already exists.
my_dict = {'a': 1, 'b': 2}
my_dict.setdefault('c', 3)
print(my_dict)
In the above code, add the key ‘c‘ with the value 3 to my_dict by using the setdefault() method. If the key ‘c‘ already existed, its value would not be changed.
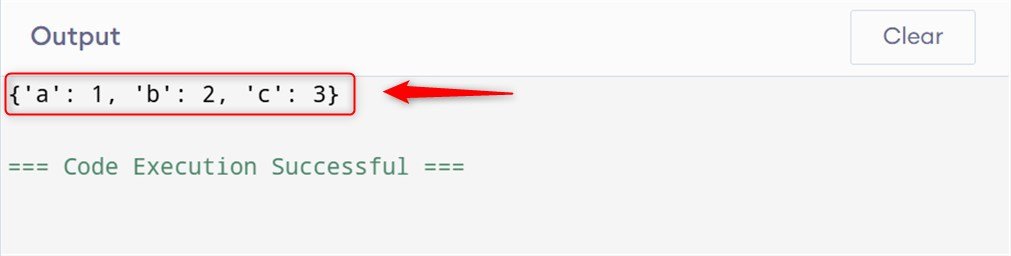
Conclusion
In Python, you can add new entries to a dictionary by using the update() function or the assignment operator. They add a new key-value pair directly.
Whether you prefer the simplicity of the assignment operator, the versatility of the update() method, the conciseness of dictionary comprehension, or the conditional addition of setdefault(), Python provides the tools you need to handle this task efficiently.