If you are worry and thinking about checking variable exists or not in Python. It is the right place to answer this query.
When working with Python, it’s often useful to know whether a variable exists before attempting to use it. It prevents errors and makes the code more robust.
In this guide, we’ll explore several methods to check if a variable exists in Python
Let’s start the guide.
4 Quick Ways to Check if Variable Exists in Python
Checking if a variable exists in Python is a common task that can be accomplished in several ways.
Let’s check the existence of variable in Python via below methods:
Method 1: Using the locals() Function
The locals() function returns a dictionary of the current local symbol table, which includes all local variables. You can use this function to check if a variable exists by looking for its name in the dictionary.
Let’s see a example:
if 'variable_name' in locals():
print("Variable exists")
else:
print("Variable does not exist")
In this example, replace ‘variable_name‘ with the name of the variable you want to check. If the variable exists, the code prints “Variable exists“; otherwise, it prints “Variable does not exist.”
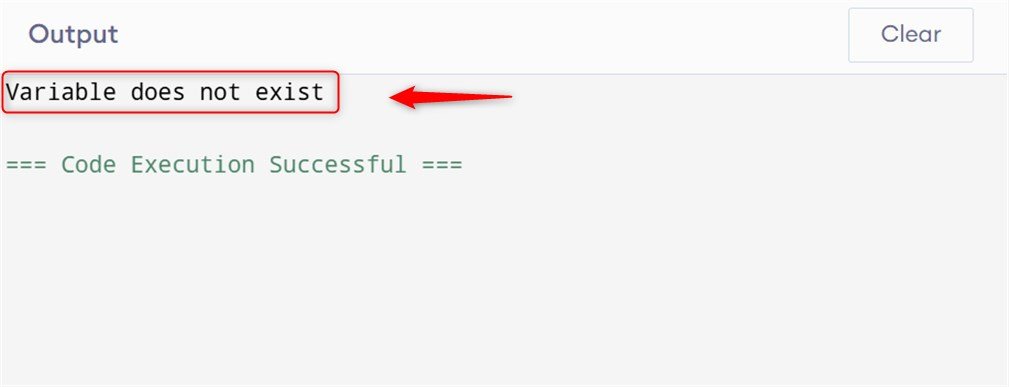
Method 2: Using the globals() Function
Similar to locals(), the globals() function returns a dictionary of the current global symbol table. It includes all global variables.
Let’s use this function:
if 'variable_name' in globals():
print("Variable exists")
else:
print("Variable does not exist")
Now, first of all, replace ‘variable_name‘ with the name of the global variable you want to check. This method is useful when you need to verify the existence of variables defined at the global scope.
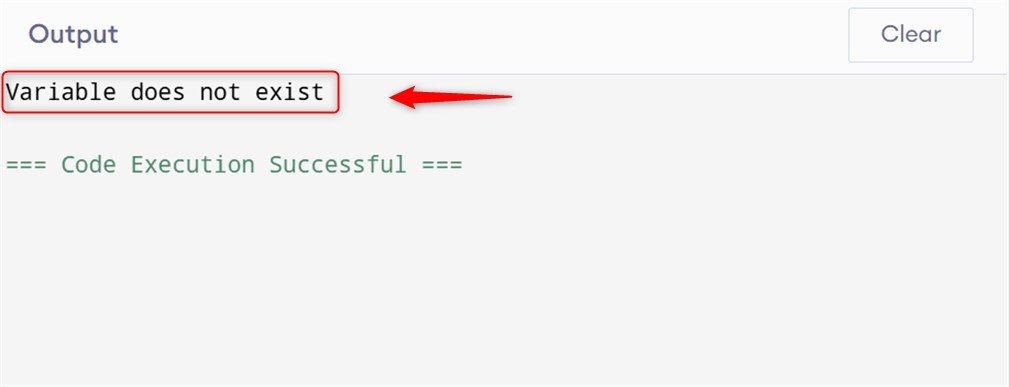
Method 3: Using a Try-Except Block
A try-except block can also be used to determine whether a variable exists. This method attempts to access the variable and catches a NameError if the variable does not exist.
Let’s see the example below:
try:
variable_name
print("Variable exists")
except NameError:
print("Variable does not exist")
In this example, replace variable_name with the name of the variable you want to check. If the variable exists, the code prints “Variable exists“; otherwise, it catches the NameError and prints “Variable does not exist.”
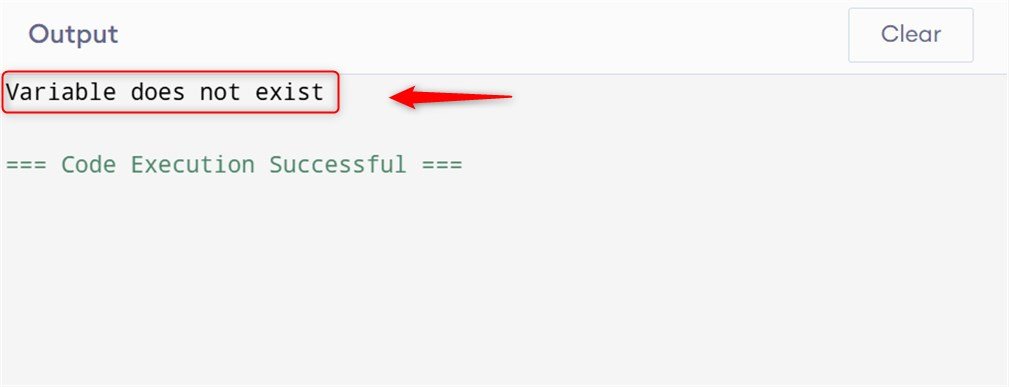
Method 4: Using the vars() Function
The vars() function returns the dict attribute of a module, class, instance, or any other object with a dict attribute.
Let’s check the variable existence in a particular scope:
if 'variable_name' in vars():
print("Variable exists")
else:
print("Variable does not exist")
In the above code, you can replace ‘variable_name‘ with the name of the variable. This method is similar to using locals() and globals(), but it can be applied to other objects as well.
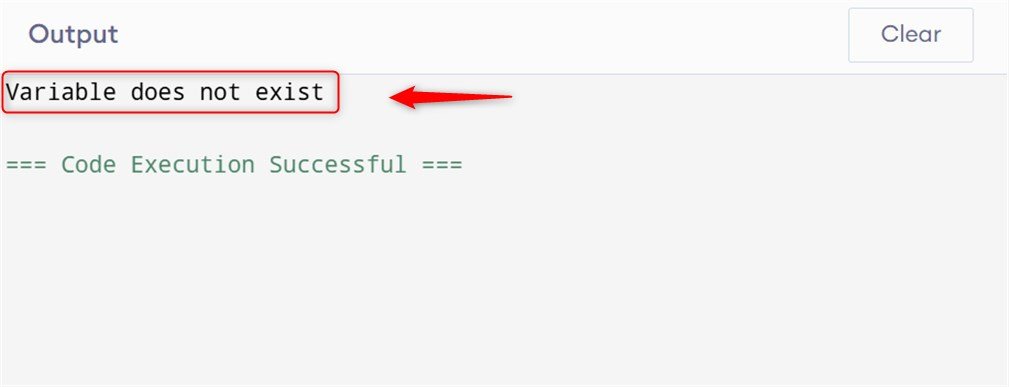
That is all from the guide.
Conclusion
To determine if a variable exists in Python, use the locals() or globals() functions to see if the variable name is in the corresponding dictionary, or use a try-except block to catch a NameError.
Whether you prefer using the locals() and globals() functions, a try-except block, or the vars() function, Python provides the tools you need to handle this task efficiently.