If you are worried about creating a GUI-based application via Python. No need to worry at all! We are here to create a GUI application using Python.
Python allows developers to build user-friendly interfaces and GUI applications that make software accessible to non-programmers. More specifically, with GUI applications, you can interact with the software through buttons, menus, and input fields, rather than relying on command-line instructions.
Let’s create a GUI-based application using Python.
How to Create a GUI Application Using Python?
I am going to create a “Quotes App” to demonstrate the process of creating a GUI application in Python. For this purpose, I will utilize the Tkinter library. Let’s begin the creation:
Step 1: Import Required Libraries
As the first step, open up your Python IDE and import the necessary libraries:
import tkinter as tk
import random
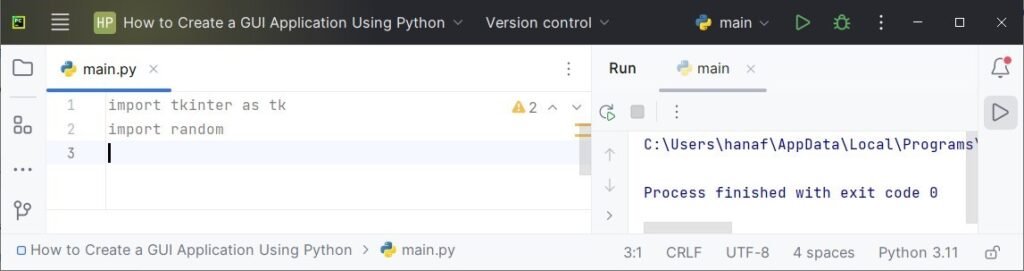
Step 2: Create a List of Quotes on the GUI Application
Next, create a list and assign it a few quotes:
quotes = [
"Growth begins where comfort ends.",
"Dreams don’t work unless you do.",
"Growth begins where comfort ends.",
"Dreams don’t work unless you do.",
]
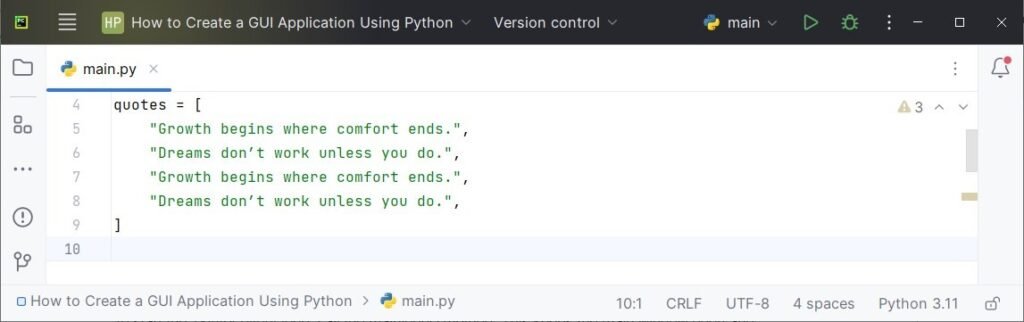
Step 3: Define a Function
After that, define a function. To select a random quote from the list, utilize the random.choice() method. The config() method is further used to update the quote_label value to the selected quote and display the quote.
def display_quote():
quote = random.choice(quotes)
quote_label.config(text=quote)

Step 4: Create a GUI Application Using Python
The first line in the below code snippet creates the main application window and assigns it to the variable “root”. Then, assign the window a title, size, and a background color:
root = tk.Tk()
root.title("Quotes App")
root.geometry("500x350")
root.config(bg="#ffefd5")
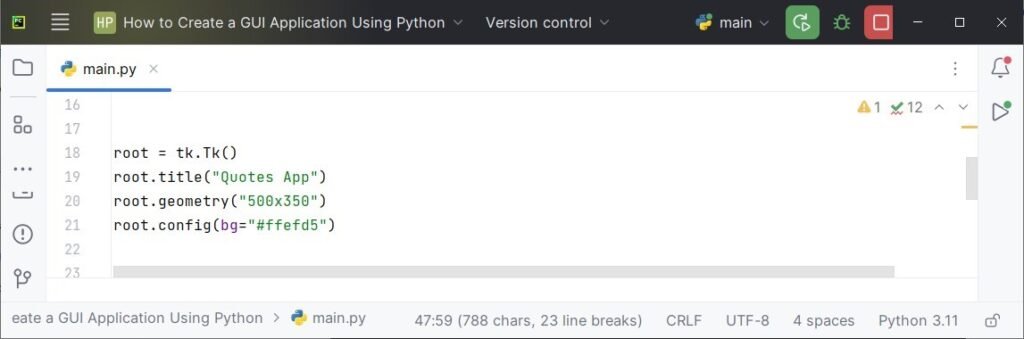

Note: To see the output of each update for the main application window, comment on the function defined above until the quote_label variable is defined.
Step 5: Create a Frame to Display Quotes Using Python
Now, create a frame to display quotes on the application’s window. Adjust the background color, border, and position:
quote_frame = tk.Frame(root, bg="#ffffff", bd=2, relief="solid")
quote_frame.place(relx=0.5, rely=0.4, anchor="center", width=450, height=200)
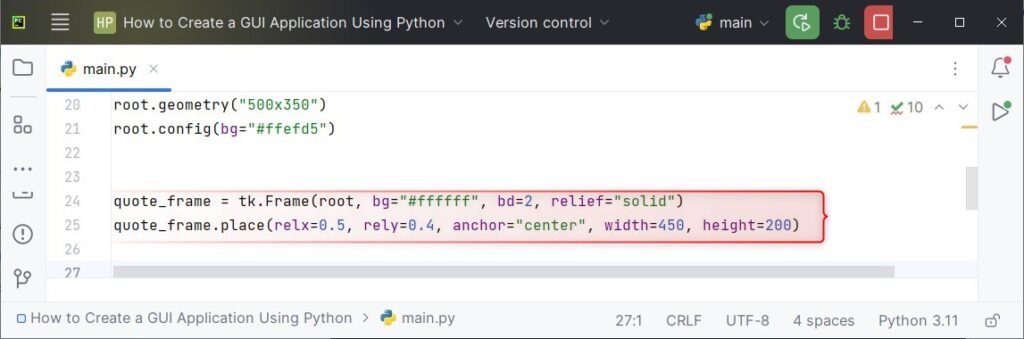
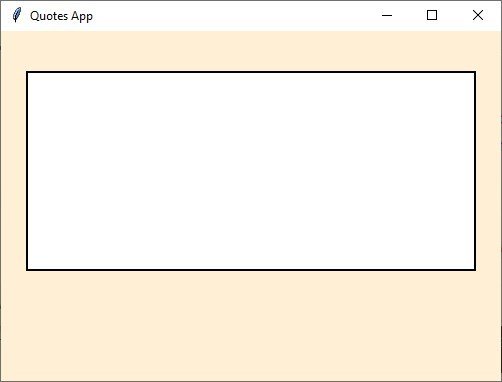
Step 6: Add a Header Label
Assign a header label to the application and apply some styles over it:
header_label = tk.Label(
root, text="Python Quotes App", font=("Helvetica", 16, "bold"),
bg="#ffefd5", fg="#333333"
)
header_label.pack(pady=10)

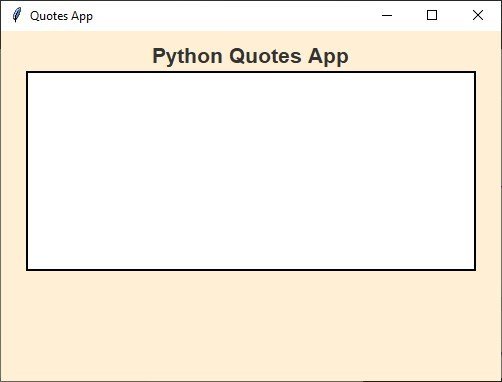
Step 7: Create a Quote Label
Next, create a quote label where the random quotes will be displayed and adjust its properties as well. Plus, uncomment the “display_quote()” function if commented earlier:
quote_label = tk.Label(
quote_frame, text="", wraplength=400, font=("Helvetica", 14, "italic"),
bg="#ffffff", fg="#333333", padx=20, pady=30
)
quote_label.pack(pady=10)
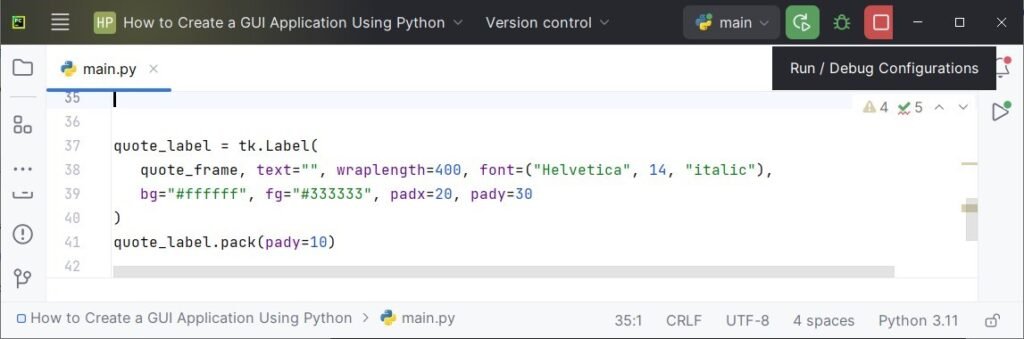
Step 8: Create a Button on a GUI Application
To display a random quote on each click, add a button to the main window:
quote_button = tk.Button(
root, text="Show Random Quote", command=display_quote,
font=("Helvetica", 12, "bold"), bg="#4CAF50", fg="white",
padx=20, pady=10, bd=0, activebackground="#45a049", cursor="hand2"
)
quote_button.place(relx=0.5, rely=0.85, anchor="center")

Step 9: Start the Tkinter Event Loop Using Python
Call the mainloop() method to keep the main window open and responsive to user actions:
root.mainloop()
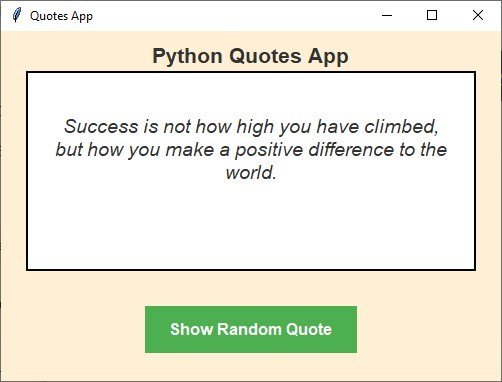
Here is the complete code:
import tkinter as tk
import random
quotes = [
"Growth begins where comfort ends.",
"Dreams don’t work unless you do.",
"Growth begins where comfort ends.",
"Dreams don’t work unless you do.",
]
root = tk.Tk()
root.title("Quotes App")
root.geometry("500x350")
root.config(bg="#ffefd5")
quote_frame = tk.Frame(root, bg="#ffffff", bd=2, relief="solid")
quote_frame.place(relx=0.5, rely=0.4, anchor="center", width=450, height=200)
header_label = tk.Label(
root, text="Python Quotes App", font=("Helvetica", 16, "bold"),
bg="#ffefd5", fg="#333333"
)
header_label.pack(pady=10)
def display_quote():
quote = random.choice(quotes)
quote_label.config(text=quote)
quote_label = tk.Label(
quote_frame, text="", wraplength=400, font=("Helvetica", 14, "italic"),
bg="#ffffff", fg="#333333", padx=20, pady=30
)
quote_label.pack(pady=10)
quote_button = tk.Button(
root, text="Show Random Quote", command=display_quote,
font=("Helvetica", 12, "bold"), bg="#4CAF50", fg="white",
padx=20, pady=10, bd=0, activebackground="#45a049", cursor="hand2"
)
quote_button.place(relx=0.5, rely=0.85, anchor="center")
root.mainloop()
Conclusion
To create a GUI application using Python, import the required libraries. Then, define all necessary variables and functions. After that, create the main application window and add labels, buttons, and styling as per your requirements.