Suppose you are worried about getting the last element of the list in Python. This blog will answer your query.
If you are working with a list of numbers, strings, or objects, you need to access the last element of a list.
This tutorial will demonstrate possible methods to get the last element of a list in Python.
5 Ways to Get the Last Element of a List in Python
Getting the last element of a list in Python is a common task that can be accomplished in several ways.
Let’s explore one by one:
Method 1: Using Negative Indexing
One of the simplest and most intuitive ways to get the last element of a list in Python is by using negative indexing. In Python, negative indices allow you to access elements from the end of the list, with -1 representing the last element.
Let’s see an example:
my_list = [1, 2, 3, 4, 5]
last_element = my_list[-1]
print(last_element)
In the above code, my_list[-1] returns the last entry 5 in the list. Negative indexing is a powerful feature that makes accessing elements from the end of a list quick and easy.
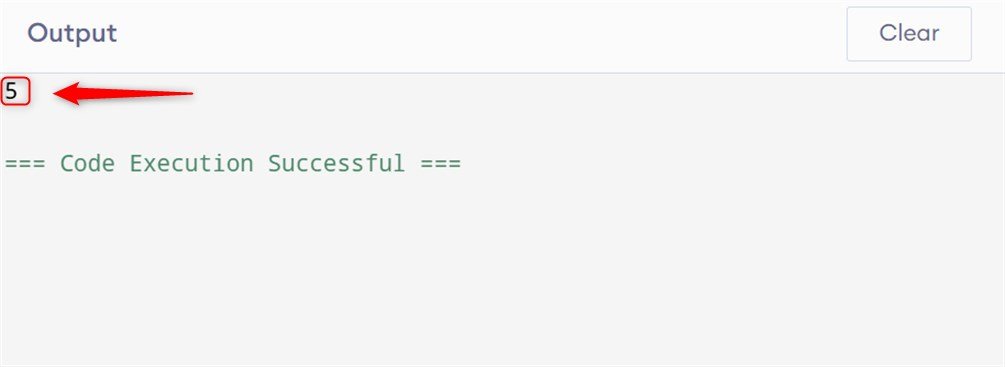
Method 2: Using the len() Function
Another way to get the last element of a list is by using the len() function. It determines the length of the list and then accessing the element at the index len(my_list) – 1:
my_list = [1, 2, 3, 4, 5]
last_element = my_list[len(my_list) - 1]
print(last_element)
In this example, len(my_list) returns the length of the list, which is 5. It is because subtracting 1 provides the last element index.
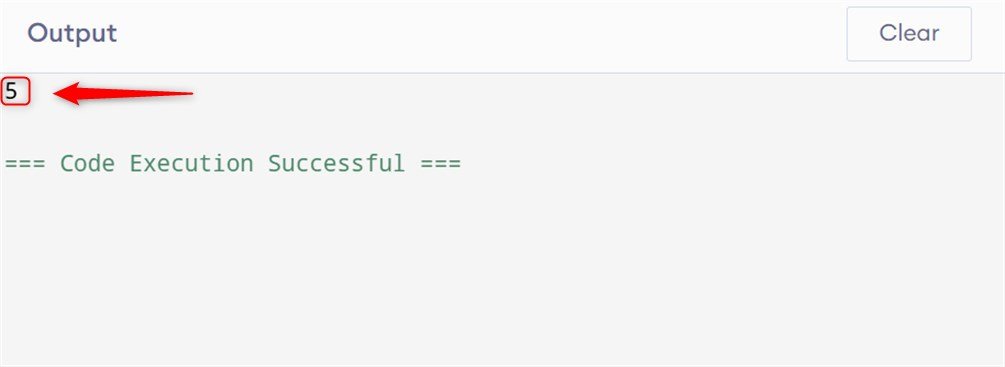
Method 3: Using the pop() Method
The pop() function can also be used to remove and return the list’s final entry. This approach is handy when you want to access and remove the last element at the same time.
Let’s see the example below:
my_list = [1, 2, 3, 4, 5]
last_element = my_list.pop()
print(last_element)
print(my_list)
Here, my_list.pop() is used to remove the last entry 5 from the list. After that, the list is updated by removing the final entry:
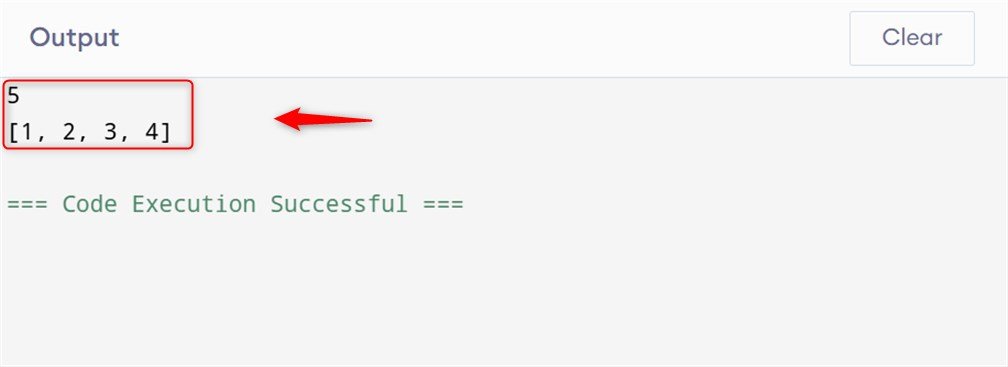
Method 4: Using Slicing
Slicing is another powerful feature in Python that allows you to access a subset of a list. By using slicing, you can get the last element of a list without modifying the original list:
my_list = [1, 2, 3, 4, 5]
last_element = my_list[-1:]
print(last_element)
In this case, my_list[-1:] returns a new list with the last entry. If you want to get the element itself, you can use my_list[-1].
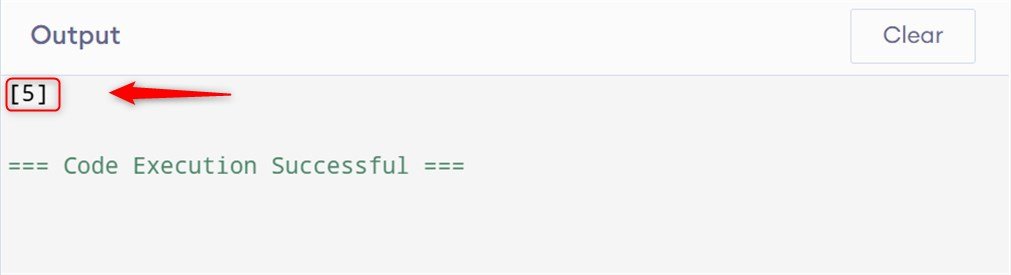
Method 5: Using the reversed() Function
Users can also get the last element of a list via the reversed() function. It returns an iterator that gets the list elements in reverse order.
Let’s use this function to get the last element by converting the iterator to a list and accessing the first element:
my_list = [1, 2, 3, 4, 5]
last_element = list(reversed(my_list))[0]
print(last_element)
In this example, reversed(my_list) returns an iterator that accesses the list elements in reverse order. After that, converting it to a list and accessing the first element gives you the last element of the original list.
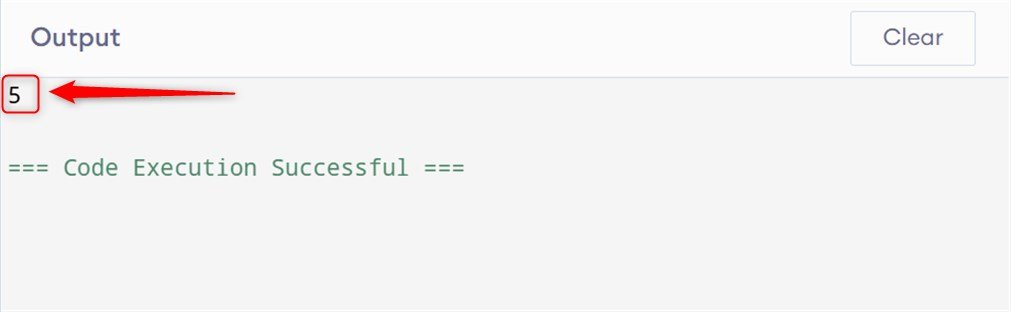
That’s it.
Conclusion
To obtain the last element of a list in Python, use negative indexing like this: my_list[-1], or use the pop() method: my_list.pop(). Both methods efficiently retrieve the last element of the list.
Whether you prefer the simplicity of negative indexing, the precision of the len() function, the dual functionality of the pop() method, the flexibility of slicing, or the power of the reversed() function, Python provides the tools you need to handle this task efficiently.