Virtual methods are one of the basic principles of object-oriented programming, which can be used to make their software very flexible and extensible. It offers a certain view to the different subclasses, though each of the subclasses is free to put as much effort into the method as it wants.
Growing your Python program can be a challenging task, this guide will explain how to put into practice virtual methods to make your program more easily maintainable and less complicated.
How to Implement Virtual Methods in Python
In Python, one will often find the use of virtual methods using the abstract base classes (ABCs) from the abc module. Virtual methods are a powerful feature in object-oriented programming paradigms; they allow the creation of software that will be easily reusable.
Understanding Virtual Methods
A virtual method is a method that is implemented in a base class but should be expected to be replaced in inherited classes. This is extremely beneficial when users have a set of related classes that share similar roles but also have unique implementations.
The step-by-step implementation of virtual methods is given below:
Step 1: Import the abc Module
To utilize virtual methods in Python, users must consider for creating a class with an abstract method through the abc prototype.
from abc import ABC, abstractmethod
Step 2: Define an Abstract Base Class
After that, users can define an abstract base class that will have the virtual method. Let’s employ the ABC class as the base class and the @abstractmethod decorator for marking the method as abstract:
class Animal(ABC):
@abstractmethod
def make_sound(self):
pass
In the above, Animal is an abstract base class with an abstract method make_sound. The pass statement refers that the function has no implementation in the base class.
Step 3: Implement the Virtual Method in Subclasses
Currently, create subclasses that inherit from the abstract base class and give their own virtual method.
class Dog(Animal):
def make_sound(self):
return "Woof!"
class Cat(Animal):
def make_sound(self):
return "Meow!"
In the above code, Dog and Cat are subclasses of Animal, and each offers its own implementation of the make_sound method.
Step 4: Utilize the Subclasses
In the end, users can create instances of the subclasses and call the virtual method:
dog = Dog()
cat = Cat()
print(dog.make_sound())
print(cat.make_sound())
The full code is provided below:
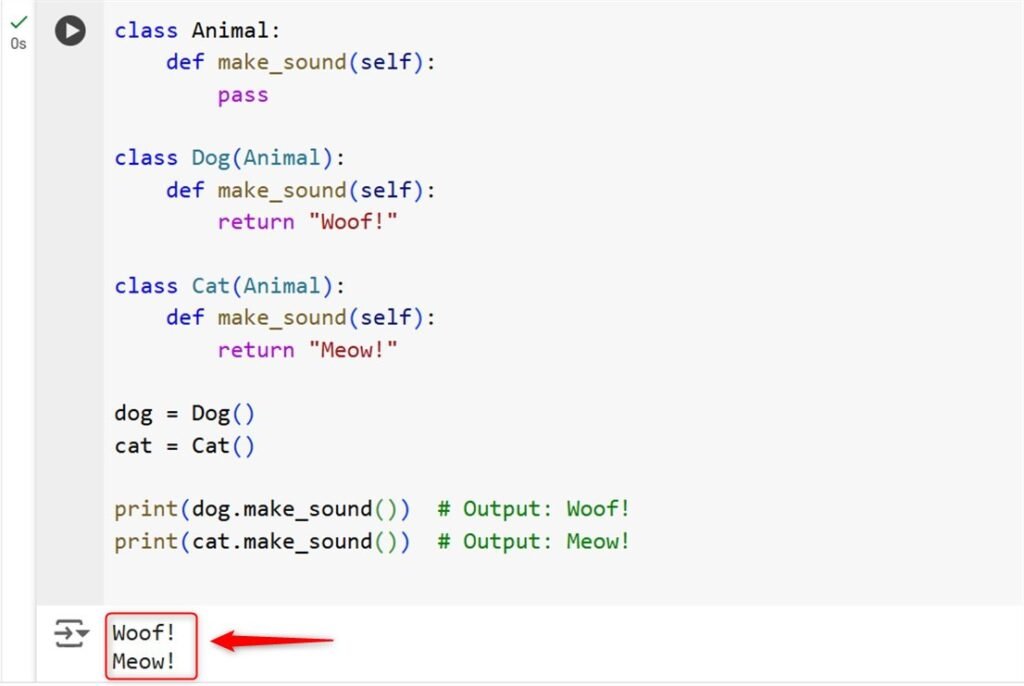
Let’s see another example.
Example: Shape Class Hierarchy
class Shape:
def area(self):
raise NotImplementedError("Subclasses must implement area()")
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14159 * self.radius * self.radius
# Polymorphic behavior
shapes = [Rectangle(2, 3), Circle(5)]
for shape in shapes:
print(shape.area())
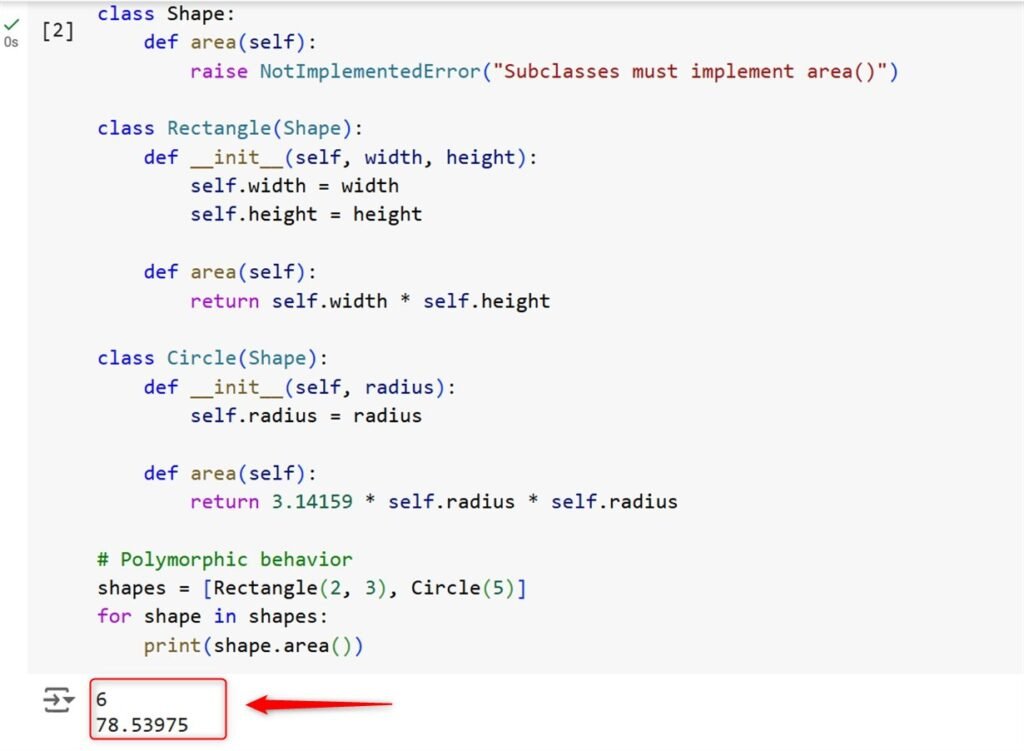
- In the above code:
- First of all, defines a virtual area() method. It forces derived classes to implement their own area() methods.
- Then, inherit from the Shape class. After that, override the area() method to calculate the area specific to their shapes.
- After that, the shapes list contains instances of both Rectangle and Circle.
Conclusion
To implement virtual methods in Python, utilizing the abc module to define an abstract base class with the @abstractmethod decorator. Subclasses then inherit from this base class and provide their own implementations of the abstract method. This approach not only enhances code reusability and flexibility but also makes your codebase more maintainable.
Keep following the engrprogrammer for more quick tutorials.