If you are thinking about “How to loop through a list in Python”, you are in the right place, we are here to fix it.
Whether you are processing data, performing calculations, or simply iterating over elements, knowing how to loop through a list efficiently is essential.
In this guide, we will explore three popular methods to loop through a list in Python.
How to Loop Through a List in Python
Looping through a list in Python is a fundamental task that can be accomplished in several ways.
Let’s explore one by one:
Method 1: Using a for Loop
The for loop is the commonly used method that allows you to access each element in the list one by one.
Let’s see an example:
my_list = [1, 2, 3, 4, 5]
for element in my_list:
print(element)
In this case, the for loop iterates through each element in my_list and outputs them. For the most common applications, this strategy is both easy and effective.
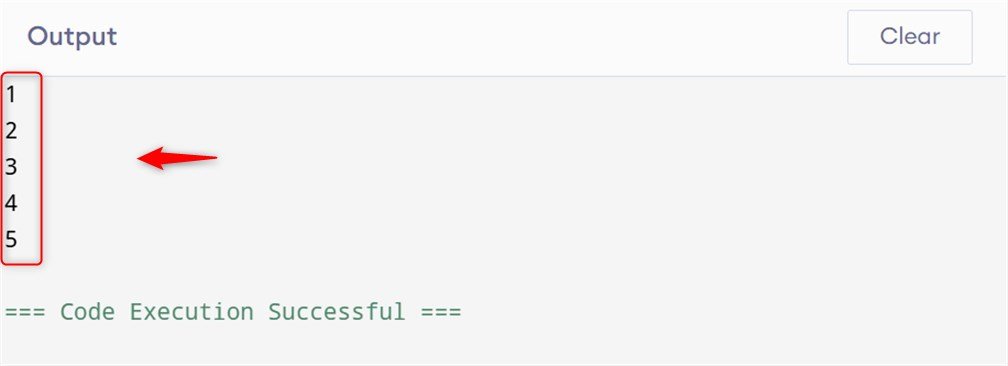
Method 2: Using enumerate() Method
The enumerate() function is a powerful tool that allows you to loop through a list while keeping track of the index of each element. This is particularly useful when you need both the element and its position in the list.
Let’s see an example:
my_list = [1, 2, 3]
for index, element in enumerate(my_list):
print(f"Index: {index}, Element: {element}")
Here, first of all, enumerate(my_list) returns the index as well as the element for each iteration. The for loop then prints the index and the element, providing more context about the position of each element in the list.
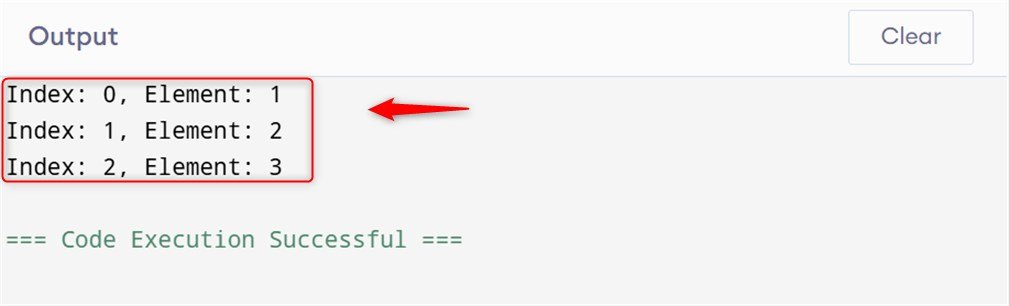
Method 3: Using List Comprehension
List comprehension is a concise and efficient way to loop through a list and perform operations on its elements. It creates a new list that depends on the existing list after implementing a particular expression to every element.
Let’s see an example below:
my_list = [1, 2, 3, 4, 5]
sq_list = [element ** 2 for element in my_list]
print(sq_list)
In this example, the list comprehension generates a new list sq_list, with each entry being the square of its corresponding element in my_list.
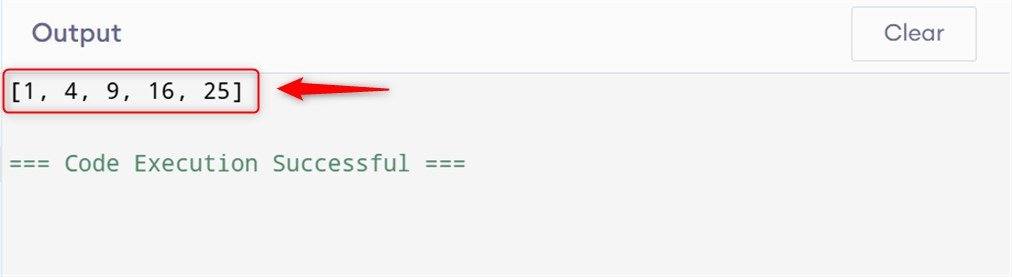
Conclusion
To loop through a list in Python, you can use a for loop for straightforward iteration, enumerate() to access both index and element or list comprehension for a concise and efficient approach.
Whether you prefer the simplicity of a for loop, the additional context provided by enumerate(), or the conciseness of list comprehension, Python offers the tools.