Are you worrying about How to Print to stderr in Python? It is the right place to answer this query!
In the world of programming, handling errors and debugging is a crucial part of the development process. One of the ways to manage this is by printing messages to stderr (standard error). However, stdout (standard output) is used for regular output.
How to Print to stderr in Python
stderr used for error messages and diagnostics. This separation allows developers to distinguish between normal output and error messages, making debugging more efficient.
This article will explore how to print to stderr in Python with several examples.
Understanding stderr and stdout
It’s essential to understand the difference between stderr and stdout. Both are file-like objects provided by the operating system:
- stdout: It utilizes regular output (e.g. print statements) (standard output stream).
- stderr: It is utilized for error messages and diagnostics (standard error stream).
By default, stdout and stderr both direct to the console. However, they redirect to different destinations, such as files or other processes. In addition, it is particularly useful for logging and debugging.
Printing to stderr in Python
In Python, printing to stderr is straightforward. Here’s how you can do it:
- Import the sys module:
import sys
- Use the print function with the file parameter:
print("This is an error message", file=sys.stderr)
This code snippet prints the message “This is an error message” to stderr instead of stdout.
Example 1: Printing Error Messages to stderr
Let’s look at a practical example where printing to stderr can be useful. Suppose you have a script that processes a list of numbers and you want to print an error message if a non-numeric value is encountered.
import sys
def process_numbers(numbers):
for number in numbers:
try:
result = int(number) * 2
print(f"Processed number: {result}")
except ValueError:
print(f"Error: '{number}' is not a valid number", file=sys.stderr)
numbers = ["10", "20", "abc", "30"]
process_numbers(numbers)
In this example, the script processes a list of numbers and prints the result to stdout. If a non-numeric value is encountered, an error message is printed to stderr. This separation ensures that error messages are easily identifiable.
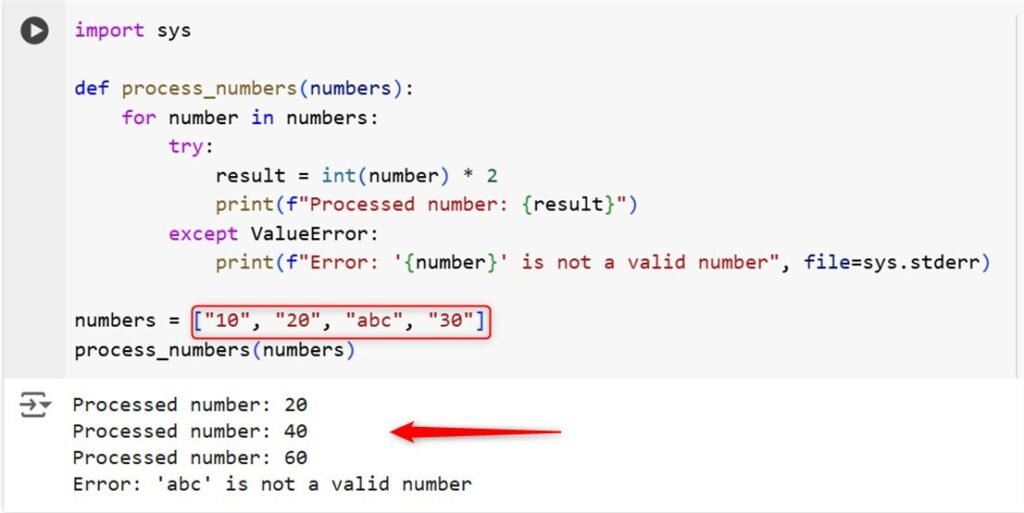
Example 2: Redirecting stderr to a File
In some cases, you might want to redirect stderr to a file for logging purposes. This occurs using the redirect_stderr context manager from the contextlib module.
import sys
from contextlib import redirect_stderr
with open('error.log', 'w') as f:
with redirect_stderr(f):
print("This is an error message", file=sys.stderr)
In this example, the error message redirects to a file named error.log instead of printing to the console. This approach is useful for logging errors in production environments where you want to keep a record of all error messages.
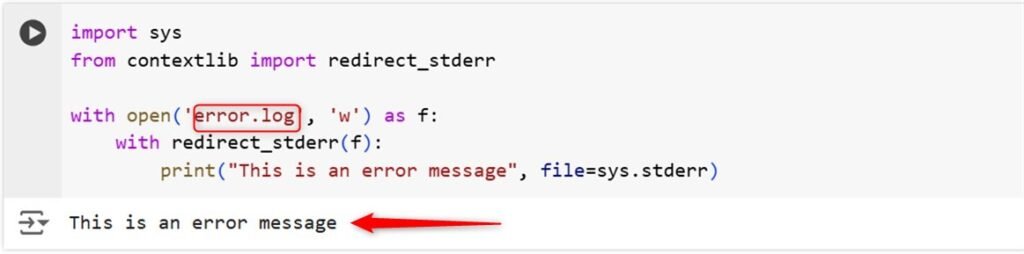
Example 3: Using a Custom Function to Print to stderr
To make your code more modular and reusable, you can create a custom function that prints messages to stderr. Here’s an example:
import sys
def print_to_stderr(message):
print(message, file=sys.stderr)
print_to_stderr("This is a custom error message")
In this example, the print_to_stderr function takes a message as an argument and prints it to stderr. This approach makes it easy to reuse the function throughout your codebase.
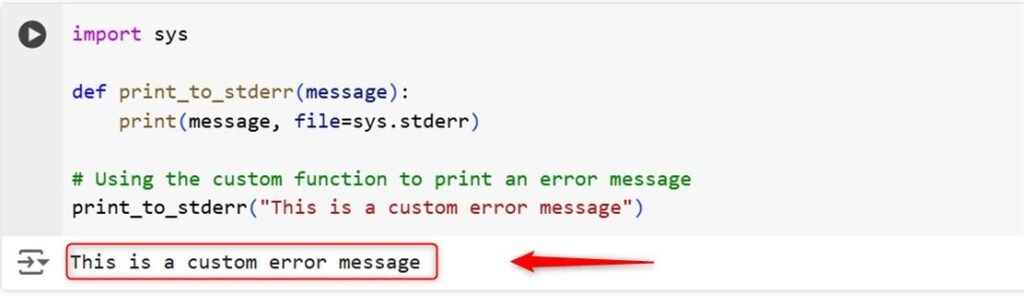
Example 4: Combining stdout and stderr Outputs
For printing messages to both stdout and stderr. Here’s how you can do it:
import sys
def process_data(data):
for item in data:
if isinstance(item, int):
print(f"Processed item: {item * 2}")
else:
print(f"Error: '{item}' is not a valid integer", file=sys.stderr)
data = [10, 20, "abc", 30]
process_data(data)
In this example, the process_data function prints processed items to stdout and error messages to stderr. This separation handles regular output and error messages appropriately.

Conclusion
Printing to stderr in Python is a simple yet powerful technique for handling errors and diagnostics. By using the sys module and the print function with the file parameter, you can easily direct error messages to stderr.
In this way, this separation of concerns enhances your ability to debug and monitor your applications effectively. Additionally, the ability to redirect stderr to files or other destinations provides flexibility for logging as well as error handling in various environments.