Are you new to Python programming? Let me tell you that the first step you’ll need to take on your coding journey is running a Python script. Don’t know where to start?
This guide will walk you through how to run a Python script in various environments, including the terminal, an IDE, and even on a Chromebook. Sounds cool? Let’s get started!
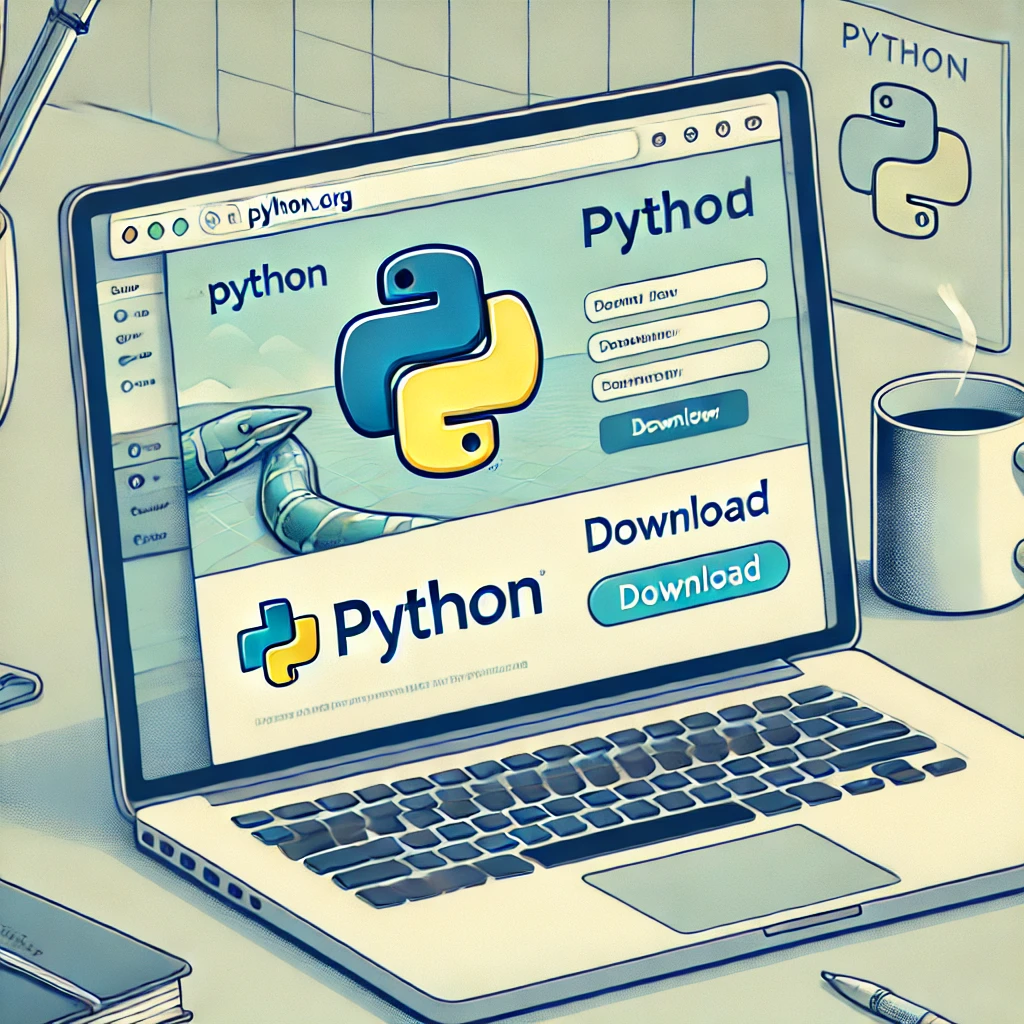
What is a Python Script?
Before we get started, let’s have a quick look on what is a Python script?
A Python script is basically a file that contains some code in the Python programming language syntax.
The scripts have the.py extension and can be run for a specific task, from simple automation to data analysis.
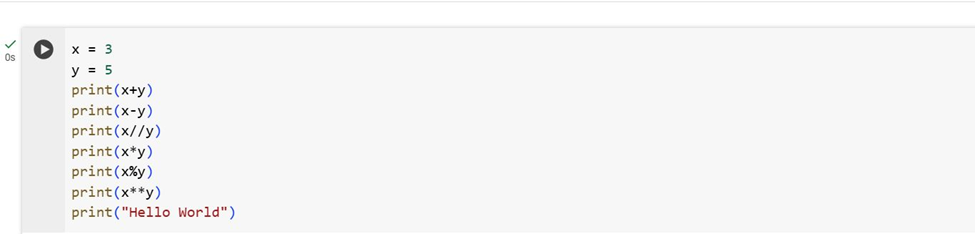
Prerequisites for Running a Python Script
To run a Python script, you’ll need:
- Python Installed on Your System
- Download the latest version of Python from python.org.
- Install Python and ensure that the Add to PATH option is selected during installation.
- A Text Editor or IDE
- Recommended IDEs include PyCharm, VS Code, or Jupyter Notebook.
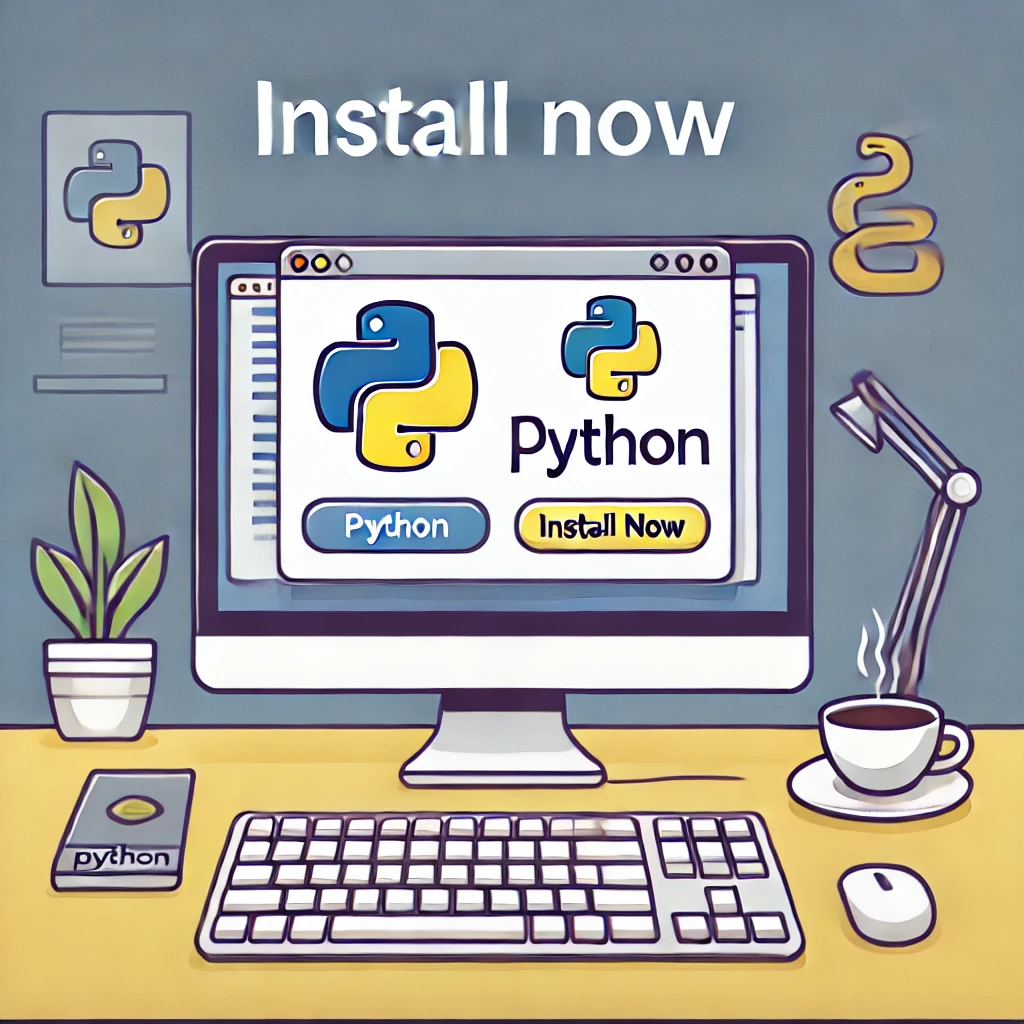
How to Run a Python Script in the Terminal
The terminal is one of the most versatile ways to run Python scripts. Follow these steps:
Step 1: Open Your Terminal
- For Windows, press Win + R, type cmd, and hit Enter.
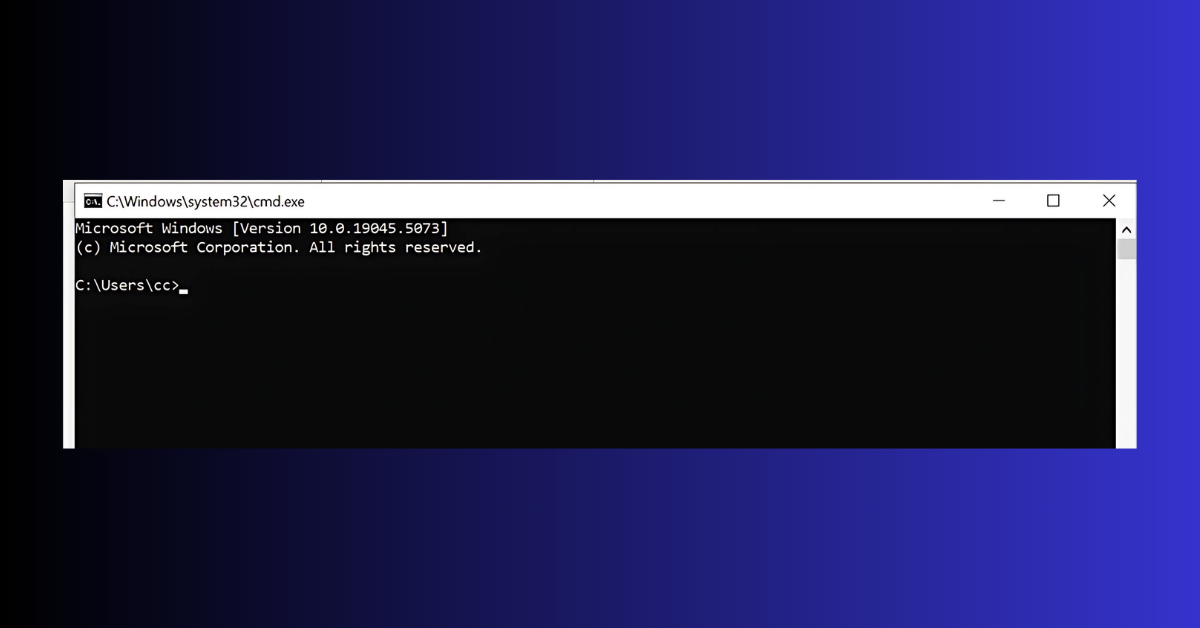
- For macOS, use Spotlight to search for “Terminal.”
- On Linux, open the default terminal.
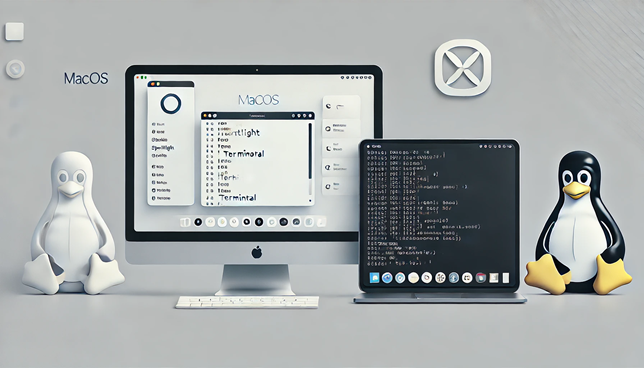
Step 2: Navigate to Your Script’s Location
Use the cd command to navigate to the folder where your Python script is saved. For example:
cd C:\Users\YourUsername\Documents\PythonScripts
Step 3: Run the Script
Enter the following command:
python script_name.py
Replace script_name.py with your actual file name.
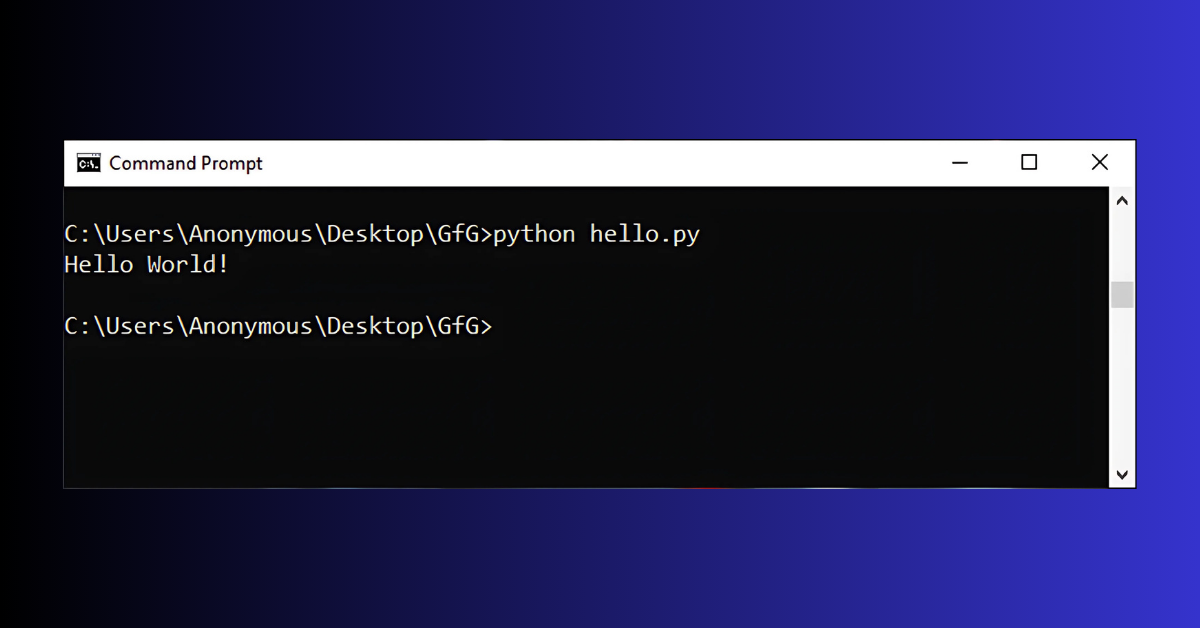
How to Run a Python Script in an IDE
If you’re working in an IDE, the process is even simpler. Here’s how to do it in PyCharm:
Step 1: Open Your Script in PyCharm
- Launch PyCharm and open the folder containing your script.
- Click on the script to open it in the editor.
Step 2: Run the Script
- Click the green play button in the top-right corner of the IDE.
Alternatively, press Shift + F10
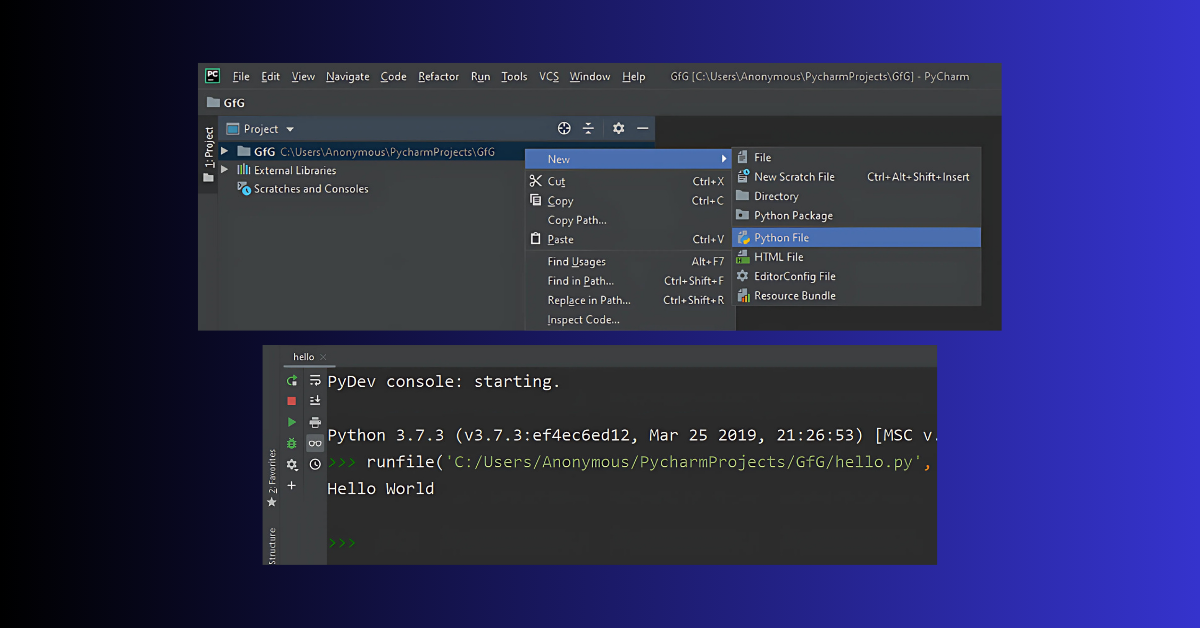
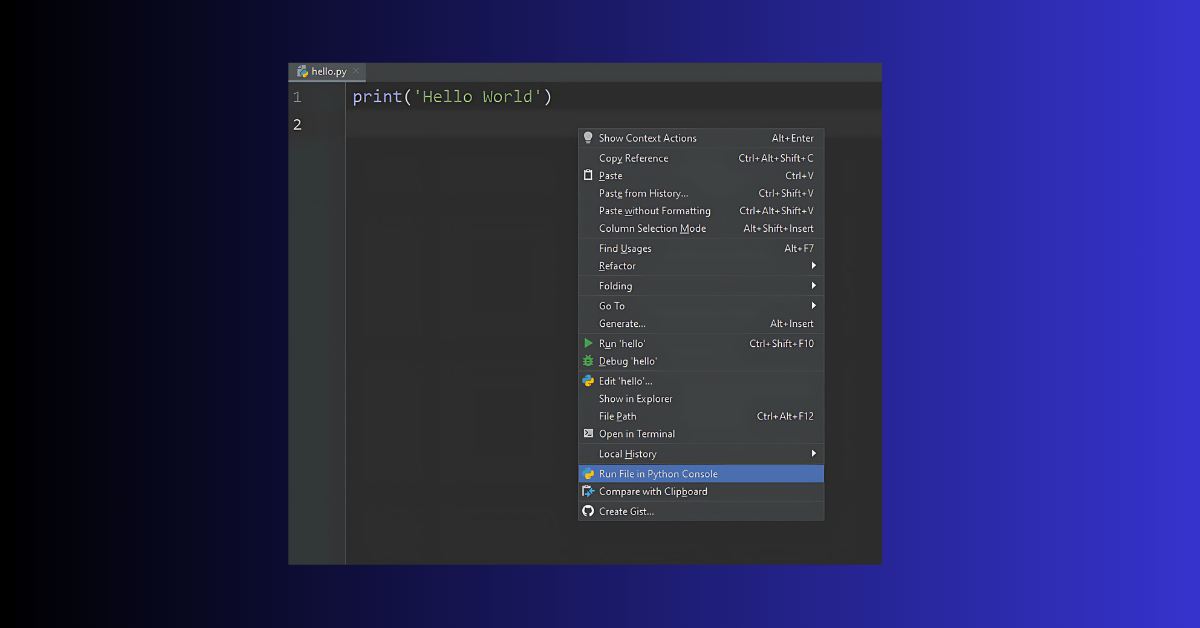
Other IDEs like VS Code and Jupyter Notebook follow similar workflows. Ensure you’ve installed Python extensions/plugins in these IDEs.
How to Run a Python Script with Multiple Input Files
Python’s power shines when processing multiple files simultaneously. Suppose you have multiple .txt files and want to analyze them in a script.
Sample Script
Here’s a script example that processes multiple input files:
import glob
# Path to input files
input_files = glob.glob(‘*.txt’)
for file in input_files:
with open(file, ‘r’) as f:
content = f.read()
print(f”Processing {file}…”)
# Add your file processing logic here
How to Run the Script
Follow the same terminal or IDE instructions as above, and ensure all input files are in the same directory as the script.
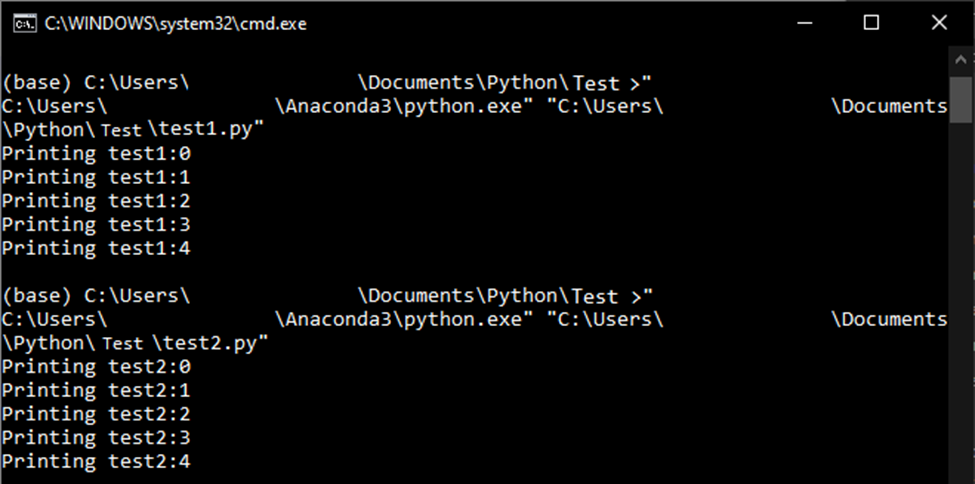
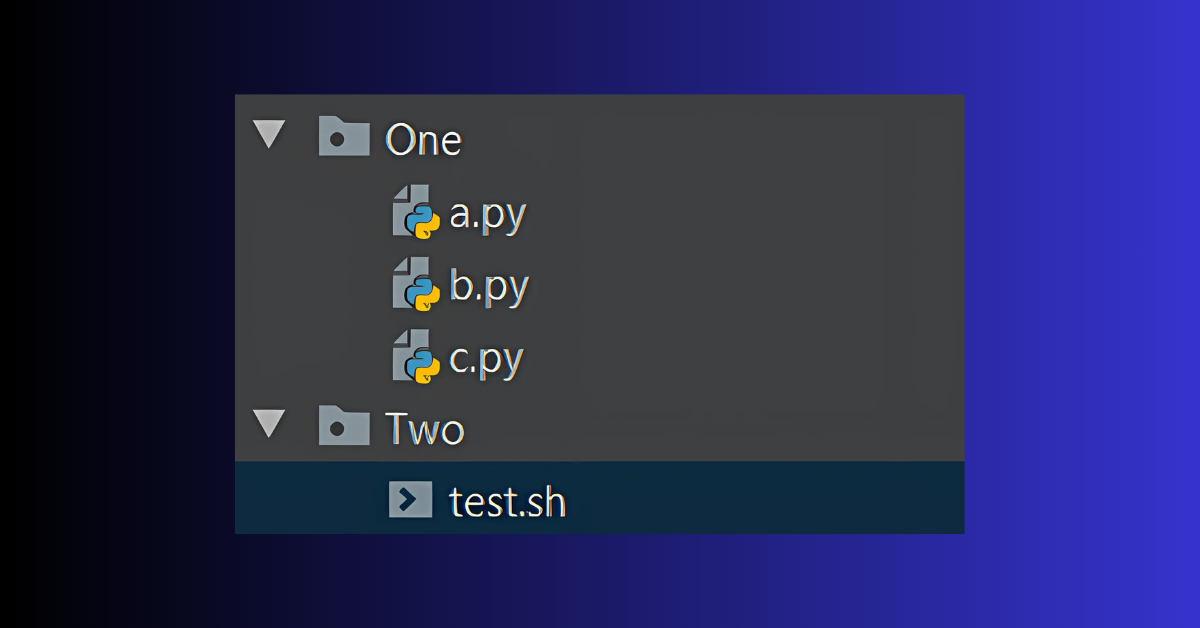
How to Run a Python Script on a Chromebook
Running Python on a Chromebook requires enabling Linux. Here’s how:
Step 1: Enable Linux on Chromebook
- Go to Settings > Developers > Enable Linux.
- Follow the prompts to install Linux.
Step 2: Install Python
- Open the Linux terminal and run:
sudo apt-get update
sudo apt-get install python3
Step 3: Run Your Script
- Save your script in the Linux Files directory.
- Use the terminal commands discussed earlier to execute it.
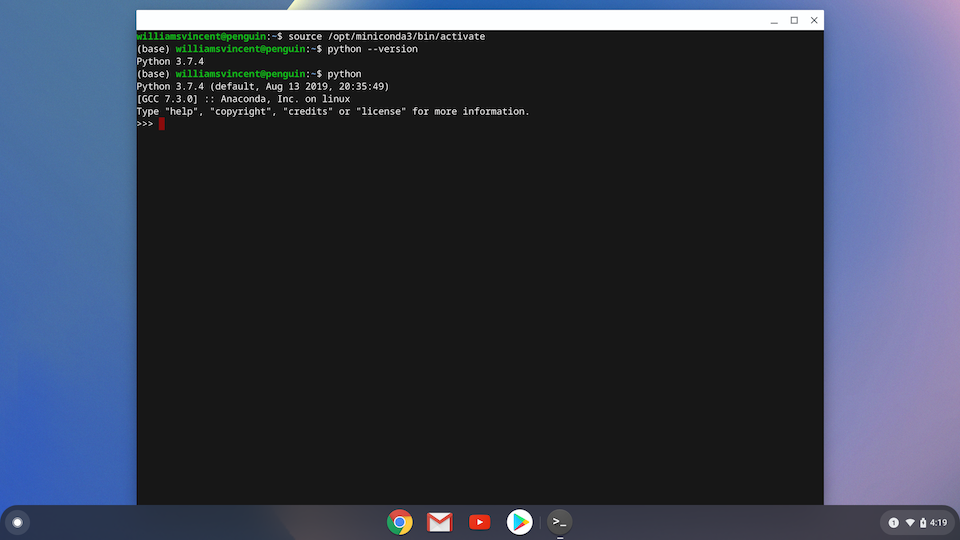
Running Python Scripts on Visual Studio Code
1: Install Visual Studio Code
- Go to the Visual Studio Code website.
- Download the installer for your operating system (Windows, macOS, or Linux).
- Install VS Code by following the on-screen instructions.
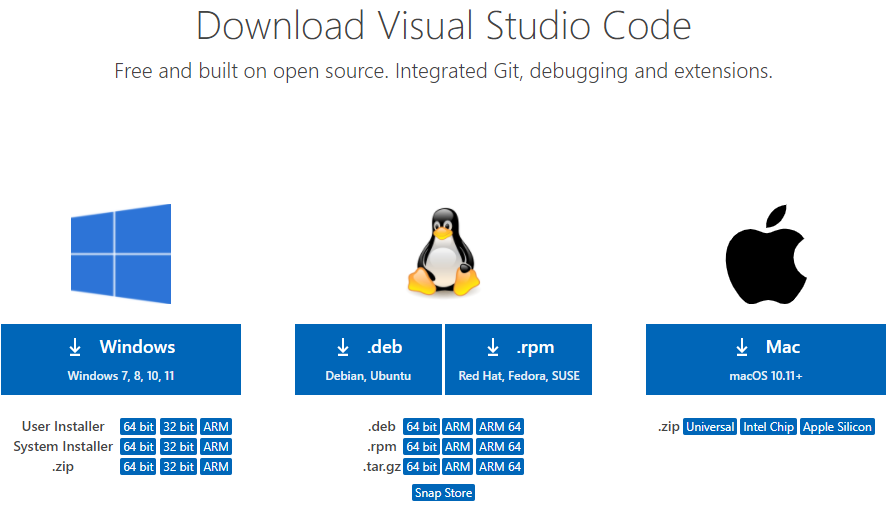
2: Install the Python Extension in VS Code
- Open VS Code.
- Navigate to the Extensions view by clicking the Extensions icon (or press Ctrl+Shift+X).
- Search for “Python” in the search bar.
- Install the official Python extension provided by Microsoft.
3: Set Up Python Interpreter
- Open VS Code and press
Ctrl+Shift+P
(orCmd+Shift+P
on macOS) to open the Command Palette. - Search for “Python: Select Interpreter”.
- Select the Python interpreter from the list (make sure it matches your Python installation).
4: Write Your Python Script
- Create a new file by clicking on File > New File.
- Save the file with a
.py
extension (e.g.,hello.py
). - Write your script, such as:
(
"Hello, World!")
5: Run Your Script
- Open the terminal in VS Code by pressing
Ctrl+`
(orCmd+
on macOS). - In the terminal, navigate to the directory where your
.py
file is saved (if not already there). - Run the script by typing:
python hello.py
6: Use the Run Button
- Alternatively, click the Run button in the top-right corner of VS Code.
- Choose Run Python File when prompted.
- The output will be displayed in the terminal below.
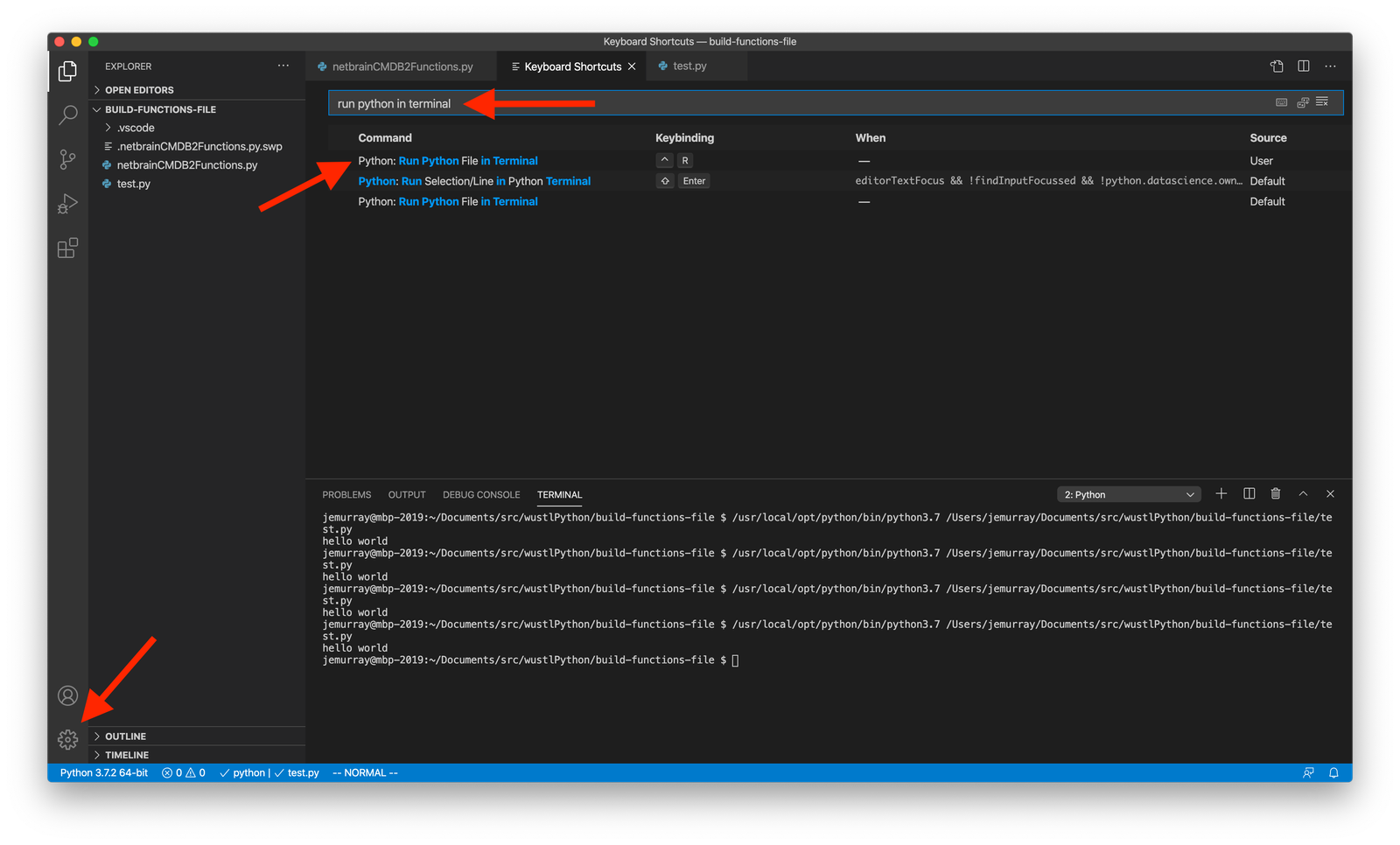
Common Issues and How to Fix Them
- ‘Python’ is Not Recognized as an Internal or External Command
- Ensure Python is added to your system’s PATH.
- Syntax Errors
- Double-check your script for typos or indentation issues.
- Module Not Found Errors
- Install the required module using:
pip install module_name
FAQs
Conclusion
Running Python scripts is straightforward once you get the hang of it.
Whether you’re using the terminal, an IDE, or a Chromebook, Python offers immense flexibility for beginners and experts alike.
Start experimenting with small scripts today to build confidence and expand your programming skills.