If you are worrying about taking integer input in Python, it is the right place to answer this query.
One of the fundamental tasks in programming is taking user input, and in many cases, this input needs to be an integer. Taking integer input is a common requirement in many programs, whether you’re building a calculator, a game, or a data processing application.
This blog will explore how to take integer input in Python, along with some practical examples and tips
How to Take Integer Input in Python?
Taking integer input in Python is a fundamental task that is essential for many applications.
To take integer input in Python, follow the below methods:
Using the input() Function
In Python, the input() function takes user input. By default, the input() function returns the input as a string. For converting this input to an integer, you can use the int() function.
Here’s a simple example:
# Taking integer input from the user
user_input = input("Enter an integer: ")
integer_input = int(user_input)
print(f"You entered the integer: {integer_input}")
In this example, the input() function prompts the user to enter a value. Then, it converts to an integer using the int() function. Finally, the converted integer stores the variable integer_input and prints it to the console.
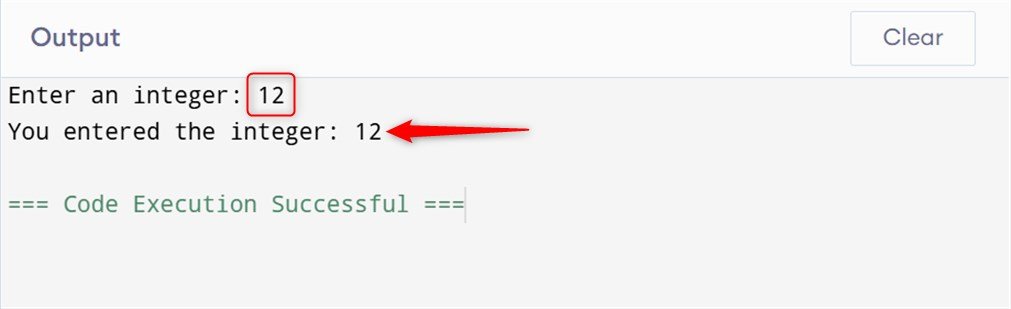
Handling Invalid Input
One of the challenges of taking integer input is handling cases where the user enters a non-integer value. To prevent your program from crashing, you can use a try-except block to catch exceptions and prompt the user to enter a valid integer.
Here’s an example:
while True:
try:
user_input = input("Enter an integer: ")
integer_input = int(user_input)
break
except ValueError:
print("Invalid input. Please enter a valid integer.")
print(f"You entered the integer: {integer_input}")
For instance, the while loop continues to prompt the user for input until a valid integer is entered. After that, if the user enters a non-integer value, the ValueError exception is caught, and an error message is displayed. Finally, the loop then repeats and allows the user to try again.
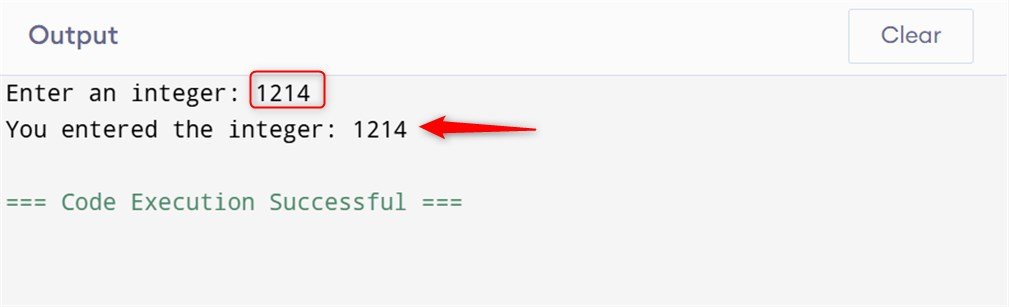
Using a Function to Take Integer Input
To make your code more modular and reusable, you can create a function that takes integer input and handles invalid input.
Here’s an example:
def get_integer_input(prompt):
while True:
try:
user_input = input(prompt)
return int(user_input)
except ValueError:
print("Invalid input. Please enter a valid integer.")
# Using the function to take integer input
integer_input = get_integer_input("Enter an integer: ")
print(f"You entered the integer: {integer_input}")
In this example, the get_integer_input function takes a prompt as an argument and returns a valid integer input. Also, the function uses a while loop and a try-except block to handle invalid input. In this way, it ensures that the user is prompted until a valid integer is entered.
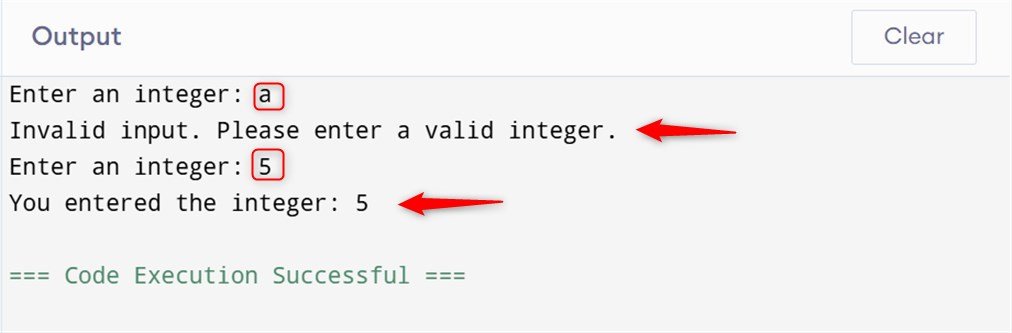
Conclusion
By using the input() and int() functions, along with try-except blocks, you can ensure that your program handles user input correctly and robustly.
Finally, ensuring that the input is an integer helps prevent errors and ensures that your program can perform the necessary calculations or operations correctly.