You do not need to worry about using the if-else statement in a single line in Python. We are here to fix it!
In Python, the ternary operator is one of the features that contribute to the if-else statement. It writes concise conditional expressions using the inline if-else statement. This allows you to evaluate conditions and execute code based on the result, all in a single line.
This article will explore the usage of the if-else statement in a single line in Python.
Understanding the Inline If-Else Statement
The inline if-else statement in Python allows you to evaluate a condition and return one of two values based on the result.
The syntax is straightforward:
value_if_true if condition else value_if_false
This expression evaluates the condition. It returns value_if_true if the condition is True, and value_if_false otherwise.
Using Inline If-Else with Print
You can use the inline if-else statement directly within the print() function to conditionally print different values.
Here’s a basic example:
x = 10
print("x has the greater value than 8" if x > 8 else "x is 8 or less")
Here, first, evaluate the condition x > 8. After that, if x is 10 is greater than 8, the expression returns “x has the greater value than 8“, finally, this string is printed.
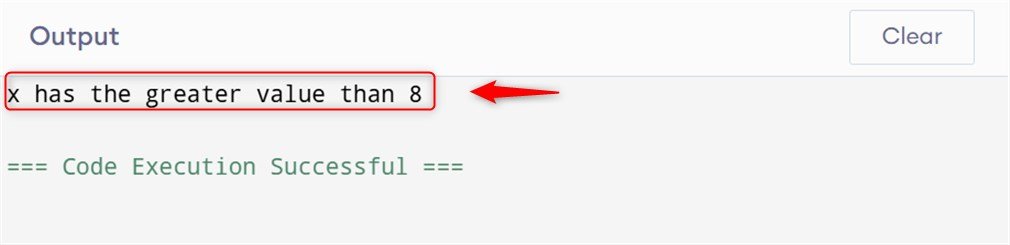
Practical Examples
Let’s look at a few practical examples to see how inline if-else statements assist in different scenarios:
1. Checking User Input
In Python, you can also check user input through the if-else statement in a single line.
Let’s see an example:
user_input = input("Enter a number: ")
print("The input number is positive" if int(user_input) > 0 else "The input number is non-positive")
In the above code, first, enter a number. The inline if-else statement checks if the entered number is positive and prints an appropriate message.
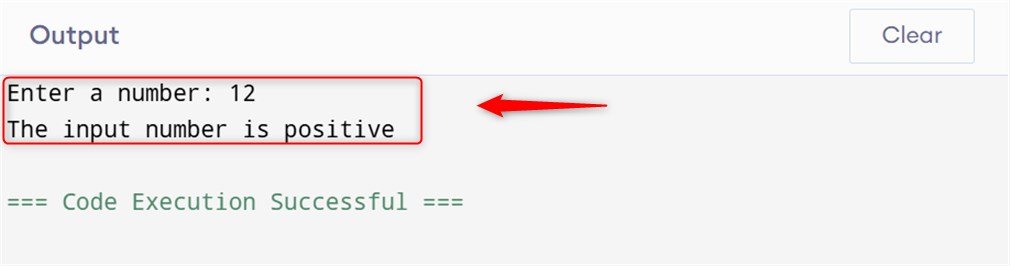
2. Conditional Formatting
Here, the inline if-else statement checks conditional formatting:
score = 85 # User Input
print("The student is Passed" if score >= 50 else " Bad Luck! The student is Failed")
Here, the inline if-else statement checks if the score is greater than or equal to 50. If it is, it prints “The student is Passed“; otherwise, it prints “Bad Luck! The student is Failed“.
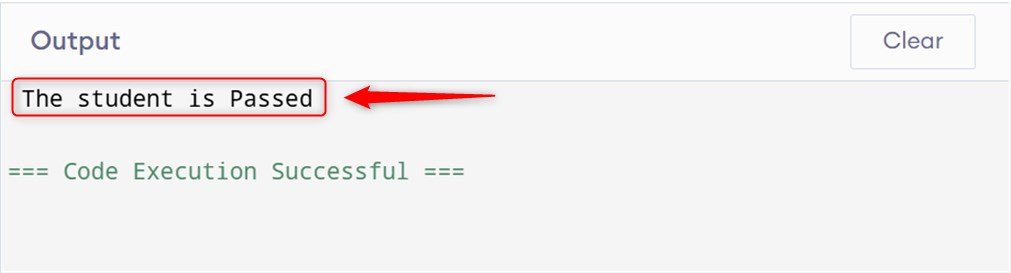
3. Handling Optional Values
In addition, the inline if-else statement checks optional values. For instance, see an example below:
name = None
print(name if name else "No name provided")
In the above code, the inline if-else statement checks if the name variable is None. If it is, it prints “No name provided“; otherwise, it prints the value of the name.
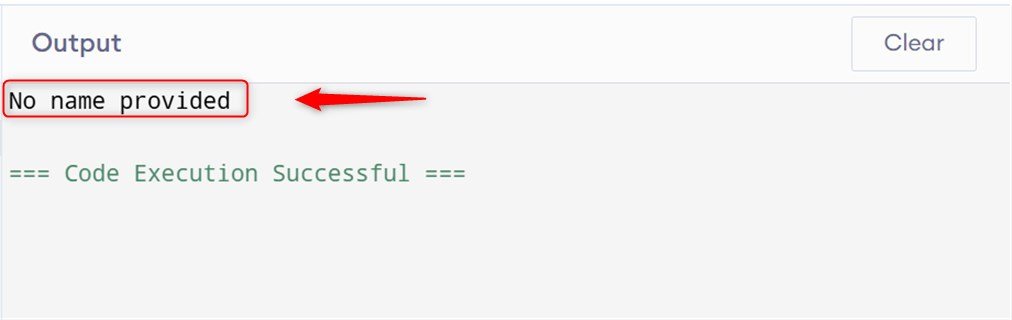
Using Inline If-Else in Assignments
You can also use inline if-else statements that can assign values to variables based on a condition.
Let’s see an example:
input_age = 20
class = "Adult" if input_age >= 15 else "Minor"
print(class)
In the above code, the class variable assigns the value “Adult” if the age is greater than or equal to 15; otherwise, it is assigned the value “Minor“.
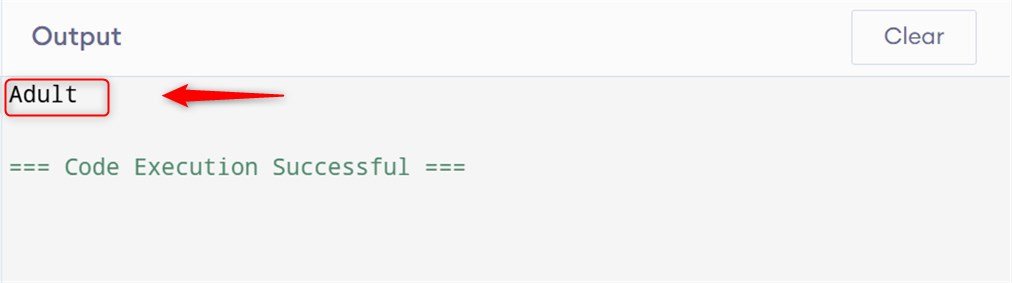
Finally, this is all from this guide.
Conclusion
In Python, use the ternary operator to use an if-else statement in a single line like this: value_if_true if condition else value_if_false. This concise syntax evaluates the condition and returns the appropriate value based on the result.
The inline if-else statement provides a flexible solution, whether you are checking user input or formatting output. Furthermore, handling optional values, or assigning variable values.