Are you thinking about the scope and lifetime of a variable in Python? We are here to answer your query!
Understanding the scope and lifetime of variables in Python is essential for writing efficient and maintainable code. By managing variable scope effectively, you can avoid common pitfalls and ensure that your code behaves as expected.
What is the Scope and Lifetime of a Variable in Python?
The scope and lifetime of variables determine where a variable can be accessed and how long it exists in memory. In Python, these principles are straightforward yet essential for writing efficient and bug-free code.
Let’s start the guide on the scope and lifetime of variables in Python.
Understanding Variable Scope
The scope of a variable refers to the area of code where it can be accessed. Python has four major forms of scope:
- Local Scope: If variables are declared inside a function means a local scope. They are only available from within that function.
- Enclosing Scope: This applies to nested functions. A variable defined in a parent function is accessible within its child functions.
- Global Scope: Variables declared at the top level of a script or module have a global scope. They are available throughout the script or module.
- Built-in Scope: These are special variables that Python reserves for its built-in functions and libraries.
Example of Variable Scope
def outer_function():
outer_var = "I'm outside!"
def inner_function():
inner_var = "I'm inside!"
print(outer_var) # Accessible
print(inner_var) # Accessible
inner_function()
print(outer_var) # Accessible
# print(inner_var) # Not accessible, would raise an error
outer_function()
In this case, outer_var has an enclosing scope, which allows it to be accessed within inner_function. However, inner_var has a limited scope and can only be accessed within inner_function.
Understanding Variable Lifetime
Lifetime means the time period a variable stores in memory. In Python, the lifetime of a variable depends on its scope:
- Local Variables: These exist only during the execution of the function in which they are defined. They are destroyed when the function exits.
- Global Variables: These exist for the duration of the program’s execution. They are formed when the program starts and destroyed when it terminates.
- Built-in Variables: These exist as long as the Python interpreter is running.
Example of Variable Lifetime
Here are some examples to illustrate the concept of variable lifetime in Python:
Example 1: Local Variable Lifetime
Let’s see an example:
def my_function():
local_var = "I live inside the function"
print(local_var)
my_function()
# print(local_var)
Local_var is generated when my_function is called and removed when it exits. If accessing local_var outside the function refers to an error.
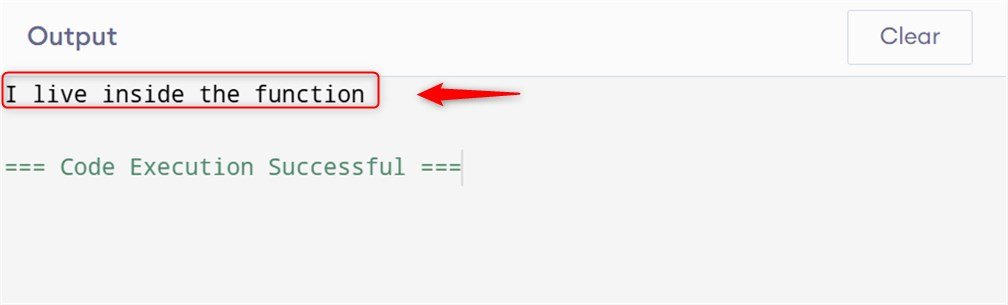
Example 2: Global Variable Lifetime
Let’s see another example:
global_var = "I live outside all functions"
def my_function():
print(global_var)
my_function()
print(global_var)
In this example, global_var is defined at the script’s top level and is accessible both within and outside of the function my_function.
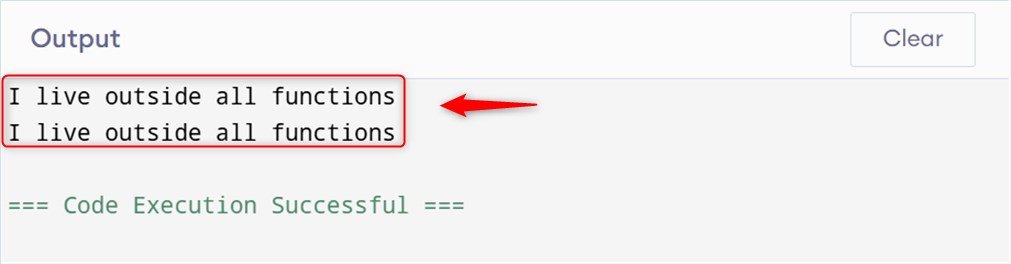
Example 3: Enclosing Variable Lifetime
Enclosing variables are created in a parent function and are accessible within its nested child functions.
def outer_function():
enclosing_var = "I live in the outer function"
def inner_function():
print(enclosing_var)
inner_function()
print(enclosing_var)
outer_function()
# print(enclosing_var)
In this example, enclosing_var is created in outer_function and is accessible within inner_function. However, it is not accessible outside outer_function.
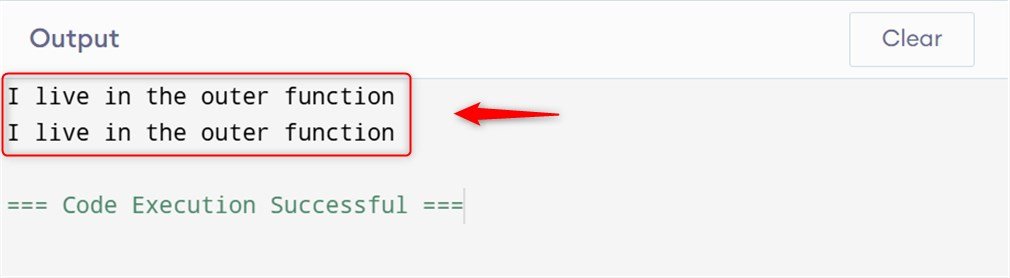
Example 4: Built-in Variable Lifetime
Built-in variables are available as long as the Python interpreter is running.
print(len("Hello, World!"))
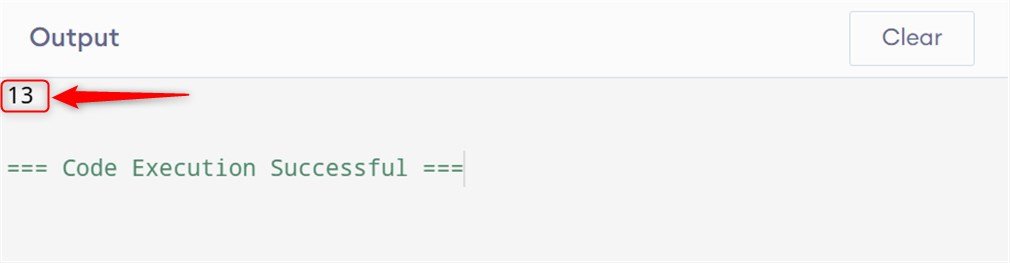
Here, a built-in function len is used for getting the length.
Best Practices for Managing Scope and Lifetime
- Use Descriptive Names: This helps avoid confusion, especially with global variables.
- Limit Global Variables: Minimize the use of global variables to reduce the risk of unintended side effects. Use local variables whenever possible.
- Avoid Nested Functions: While nested functions can be useful, overusing them can make your code harder to read and debug. Use them judiciously.
Conclusion
In Python, the scope of a variable determines where it can be accessed within the code, while its lifetime refers to how long it exists in memory. Local variables exist only within their function, whereas global variables persist throughout the program’s execution.
Remember to use descriptive names, limit global variables, encapsulate your code, and avoid excessive nesting. With these best practices, you’ll be well on your way to mastering variable scope and lifetime in Python.